怎么在Vue3里面写运行python程序
时间: 2023-12-03 09:05:51 浏览: 70
在Vue3中运行Python程序需要使用后端技术,比如Node.js和Express框架。以下是一些基本步骤:
1. 创建一个Node.js项目,并使用Express框架搭建后端服务器。
2. 安装Python和Python的包管理器pip。
3. 在Express中使用child_process模块运行Python程序。
4. 将Vue3项目中的请求发送到后端服务器,并处理响应。
下面是一个简单的例子,可以帮助你开始:
1. 创建一个新的Node.js项目,打开终端并进入项目目录,输入以下命令:
```
npm init -y
```
2. 安装Express框架和其他必要的包:
```
npm install express child_process body-parser cors --save
```
3. 创建一个名为"app.js"的新文件,并添加以下代码:
```javascript
const express = require('express');
const cors = require('cors');
const bodyParser = require('body-parser');
const { exec } = require('child_process');
const app = express();
app.use(cors());
app.use(bodyParser.json());
app.post('/runPython', (req, res) => {
const { code } = req.body;
exec(`python -c "${code}"`, (error, stdout, stderr) => {
if (error) {
res.status(500).json({ message: error.toString() });
} else if (stderr) {
res.status(500).json({ message: stderr });
} else {
res.status(200).json({ message: stdout });
}
});
});
app.listen(3000, () => console.log('Server started on port 3000'));
```
4. 在Vue3项目中,创建一个新的文件并添加以下代码:
```javascript
import axios from 'axios';
export default {
methods: {
async runPython(code) {
try {
const response = await axios.post('http://localhost:3000/runPython', { code });
console.log(response.data.message);
} catch (error) {
console.error(error.response.data.message);
}
}
}
};
```
以上代码中,通过使用axios库向后端服务器发送POST请求,触发/runPython路由处理程序,并将代码发送到后端服务器以运行Python程序。在控制台中打印响应消息。
5. 在Vue3的组件中调用runPython方法,并传递Python代码作为参数。
```javascript
import { defineComponent } from 'vue';
import pythonRunner from './pythonRunner';
export default defineComponent({
setup() {
const { runPython } = pythonRunner();
const onClick = async () => {
const code = 'print("Hello, world!")';
await runPython(code);
};
return {
onClick
};
},
template: `
<button @click="onClick">Run Python</button>
`
});
```
以上代码中,在Vue3组件的setup函数中,通过调用pythonRunner方法获取runPython函数,并将其绑定到onClick方法中。最后,将onClick方法绑定到按钮的点击事件上。
请注意,此示例仅用于说明如何在Vue3中运行Python程序,实际应用中还需要考虑安全性和性能等方面的问题。
相关推荐
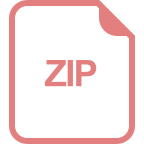
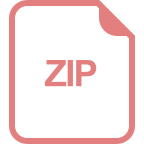
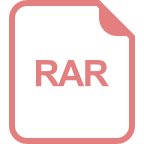














