java 枚举类型自定义排序
时间: 2023-10-14 10:34:38 浏览: 173
好的,让我为您解答。
Java 中的枚举类型可以通过实现 `Comparable` 接口来进行自定义排序。下面是一个示例代码,它演示了如何对一个枚举类型进行自定义排序:
```java
public enum Fruit implements Comparable<Fruit> {
APPLE, ORANGE, BANANA, PINEAPPLE;
@Override
public int compareTo(Fruit other) {
switch (this) {
case APPLE:
if (other == APPLE) {
return 0;
} else {
return -1;
}
case ORANGE:
if (other == APPLE) {
return 1;
} else if (other == ORANGE) {
return 0;
} else {
return -1;
}
case BANANA:
if (other == PINEAPPLE) {
return -1;
} else {
return 1;
}
case PINEAPPLE:
if (other == PINEAPPLE) {
return 0;
} else {
return 1;
}
default:
throw new IllegalArgumentException("Unknown fruit type: " + this);
}
}
}
```
在上面的示例代码中,我们定义了一个名为 `Fruit` 的枚举类型,它包含了四个水果:苹果、橙子、香蕉和菠萝。我们实现了 `Comparable` 接口,并覆盖了 `compareTo` 方法,该方法根据我们指定的顺序比较两个水果。
在 `compareTo` 方法中,我们使用了 `switch` 语句来比较两个水果。首先,我们比较了当前水果和另一个水果是否相同。如果它们相同,我们返回 0。如果它们不同,我们根据我们指定的顺序返回 -1 或 1。
最后,我们可以使用 `Arrays.sort()` 函数对枚举类型进行排序,如下所示:
```java
Fruit[] fruits = { Fruit.PINEAPPLE, Fruit.ORANGE, Fruit.APPLE, Fruit.BANANA };
Arrays.sort(fruits);
System.out.println(Arrays.toString(fruits));
```
在上面的示例代码中,我们创建了一个包含四个水果的数组,并使用 `Arrays.sort()` 函数对它们进行排序。输出结果如下:
```
[APPLE, ORANGE, BANANA, PINEAPPLE]
```
希望这可以解答您的问题。如果您有任何其他问题,请随时问我。
阅读全文
相关推荐
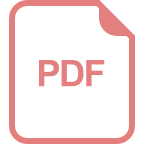
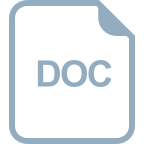
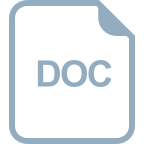
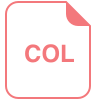
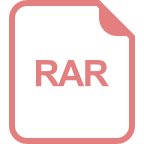
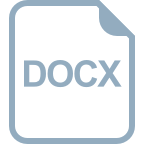
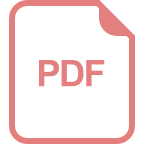
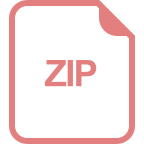
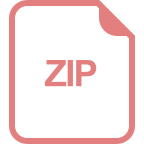
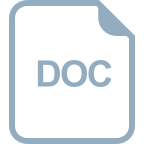
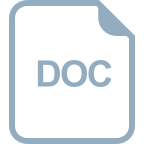
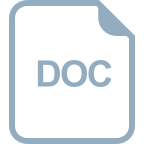
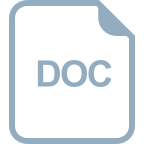
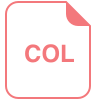
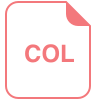
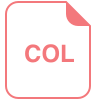