spdk_json_write_string_fmt
时间: 2023-09-10 18:12:41 浏览: 92
`spdk_json_write_string_fmt` 是 SPDK (Storage Performance Development Kit) 库中的一个函数,用于将一个格式化的字符串作为 JSON 字符串写入到 JSON 输出缓冲区中。
函数原型如下:
```c
int spdk_json_write_string_fmt(struct spdk_json_write_ctx *w, const char *fmt, ...)
```
该函数接受一个 `spdk_json_write_ctx` 结构体指针参数和一个格式化字符串参数,以及可变参数列表。 `spdk_json_write_ctx` 结构体指针参数是一个 JSON 写入上下文,它包含了一个指向输出缓冲区的指针和输出缓冲区的长度。函数将格式化字符串作为 JSON 字符串写入到输出缓冲区中,并返回写入的字符数,如果写入失败,则返回负数。
相关问题
把这段代码转化为python代码(package service import ( "encoding/json" "errors" "fmt" "gin-syudy/api/device/req" "gin-syudy/define" "gin-syudy/models" "gin-syudy/mqtt" "gin-syudy/tools/resp" "gin-syudy/utils" mq "github.com/eclipse/paho.mqtt.golang" "github.com/gin-gonic/gin" "log" "net/http" "strconv" "time" ) // DeviceController 控制设备 // @BasePath /api/v1 // @Description 启动对应设备 // @Tags 启动设备 // @param identity query string false "Identity" // @param controllerId query string false "controllerId" // @param controlState query string false "controlState" // @Success 200 {object} resp.Response "{"code":200,"data":[...]}" // @Failure 502 {object} resp.Response "{"code":502,"data":[...]}" // @Router /api/v1/device/start [Post] func DeviceController(c *gin.Context) { device := new(models.DeviceBasic) write := new(mqtt.Write) device.Identity = c.Query("identity") id, _ := strconv.Atoi(c.Query("controllerId")) fmt.Println(id) state, _ := strconv.Atoi(c.Query("controllerState")) fmt.Println(state) write.Id = uint32(id) write.State = uint32(state) if device.Identity == "" { resp.RespFail(c, http.StatusBadGateway, errors.New("必填参数为空"), resp.FoundFail) return } deviceBasic := device.GetTopicByIdentity() subTopic := "Device/" + deviceBasic.ItemName + "/" + deviceBasic.BridgeName + "/control" + deviceBasic.Secret fmt.Println(subTopic) sendTopic := "Host/" + deviceBasic.ItemName + "/" + deviceBasic.BridgeName + "/control" + deviceBasic.Secret fmt.Println(sendTopic) dataChan := make(chan *mqtt.StartDataResp, 1) mqtt.SubscribeMessage(subTopic, func(client mq.Client, message mq.Message) { fmt.Printf("MESSAGE : %s\n", message.Payload()) fmt.Printf("TOPIC : %s\n", message.Topic()) subscribeStartData := new(mqtt.StartDataResp) err := json.Unmarshal(message.Payload(), &subscribeStartData) if err != nil { resp.RespFail(c, http.StatusBadGateway, err, "回调函数格式不正确") return } dataChan <- subscribeStartData }) startData := new(mqtt.StartData) startData.SampTime = time.Now().String() startData.CommandID = utils.GetUUid() startData.Write = write data, _ := json.Marshal(startData) err := mqtt.SendMessage(sendTopic, data) if err != nil { resp.RespFail(c, http.StatusBadGateway, err, resp.FoundFail) return } responseMessage := <-dataChan err, _ = mqtt.Unsubscribe(sendTopic) if err != nil { resp.RespFail(c, http.StatusBadGateway, err, "取消订阅失败") return } resp.RespOK(c, responseMessage, "控制成功") })
以下是将给定的 Go 代码翻译为 Python 的代码:
```python
import json
import logging
import time
from http import HTTPStatus
import paho.mqtt.client as mqtt
from flask import jsonify, request
from service.models import DeviceBasic
from service.mqtt import SendMessage, SubscribeMessage, Unsubscribe
from service.tools.resp import RespFail, RespOK
from service.utils import GetUUid
# DeviceController
# 控制设备
# @BasePath /api/v1
# @Description 启动对应设备
# @Tags 启动设备
# @param identity query string false "Identity"
# @param controllerId query string false "controllerId"
# @param controlState query string false "controlState"
# @Success 200 {object} resp.Response "{"code":200,"data":[...]}"
# @Failure 502 {object} resp.Response "{"code":502,"data":[...]}"
# @Router /api/v1/device/start [Post]
def DeviceController():
device = DeviceBasic()
device.identity = request.args.get('identity', '')
id = int(request.args.get('controllerId', 0))
logging.debug(f"id: {id}")
state = int(request.args.get('controllerState', 0))
logging.debug(f"state: {state}")
write = Write()
write.id = id
write.state = state
if not device.identity:
RespFail(HTTPStatus.BAD_GATEWAY, errors=["必填参数为空"])
return
deviceBasic = device.GetTopicByIdentity()
subTopic = f"Device/{deviceBasic.ItemName}/{deviceBasic.BridgeName}/control{deviceBasic.Secret}"
logging.debug(f"subTopic: {subTopic}")
sendTopic = f"Host/{deviceBasic.ItemName}/{deviceBasic.BridgeName}/control{deviceBasic.Secret}"
logging.debug(f"sendTopic: {sendTopic}")
dataChan = []
def on_message(client, userdata, message):
logging.debug(f"MESSAGE : {message.payload}")
logging.debug(f"TOPIC : {message.topic}")
subscribeStartData = mqtt.StartDataResp()
try:
subscribeStartData = json.loads(message.payload)
except json.JSONDecodeError as e:
RespFail(HTTPStatus.BAD_GATEWAY, errors=[f"回调函数格式不正确: {str(e)}"])
return
dataChan.append(subscribeStartData)
mqtt.SubscribeMessage(subTopic, on_message)
startData = mqtt.StartData()
startData.SampTime = time.strftime("%Y-%m-%d %H:%M:%S", time.localtime())
startData.CommandID = GetUUid()
startData.Write = write
data = json.dumps(startData, default=lambda obj: obj.__dict__, ensure_ascii=False)
err = SendMessage(sendTopic, data.encode())
if err:
RespFail(HTTPStatus.BAD_GATEWAY, errors=[err])
return
responseMessage = dataChan[0]
err = Unsubscribe(sendTopic)
if err:
RespFail(HTTPStatus.BAD_GATEWAY, errors=[f"取消订阅失败: {err}"])
return
RespOK(responseMessage, message="控制成功")
```
func Exec(command string) error { in := bytes.NewBuffer(nil) cmd := exec.Command("sh") cmd.Stdin = in in.WriteString(command) in.WriteString("exit\n") if err := cmd.Run(); err != nil { return err } return nil } func (h *Headscale) GetRoutesIp(w http.ResponseWriter, r *http.Request) { w.Header().Set("Content-Type", "application/json") var req GetRoutesIpReq jsonerr := json.NewDecoder(r.Body).Decode(&req) if jsonerr != nil { http.Error(w, "Failed to decode JSON", http.StatusBadRequest) return } response := GetRoutesIpResp{} cmd := exec.Command("/root/digital_guard/headscale", "routes", "list") routesCmdString := cmd.String() output, cmderr := cmd.CombinedOutput() if cmderr != nil { log.Info().Msgf("Failed to execute shell command: %s", cmderr.Error()) response.Messge = append(response.Messge, routesCmdString) } data := string(output) lines := strings.Split(data, "\n") for _, ipToFinds := range req.Ip { for _, line := range lines { if strings.Contains(line, ipToFinds) && strings.Contains(line, req.Name) { fields := strings.Fields(line) if len(fields) >= 3 { id := fields[0] // s := []string{"/root/digital_guard/headscale routes enable --route ", "1", " set-Advertised=true set-Enabled=true"} // s[1] = id // strCmdbyte := strings.Join(s, "") // errs := Exec(strCmdbyte) errs := Exec("/root/digital_guard/headscale routes enable --route " + id + " set-Advertised=true set-Enabled=true") if errs != nil { // log.Info().Msg(fmt.Sprintf("-----------err------shell命令----------: %s", strCmdbyte)) // response.Messge = append(response.Messge, strCmdbyte) } else { response.Code = 1 } } } } } respJSON, err := json.Marshal(response) if err != nil { http.Error(w, "Failed to encode JSON", http.StatusInternalServerError) return } w.WriteHeader(http.StatusOK) w.Write(respJSON) } 这样执行的linux命令失败
根据您提供的代码,我看到您尝试执行的 Linux 命令是 `/root/digital_guard/headscale routes enable --route <id> set-Advertised=true set-Enabled=true`,您说该命令执行失败,但是没有提供具体的错误信息。如果您能够提供该命令执行失败的具体错误信息,我可以更好地帮助您解决问题。
除此之外,您的代码中使用了 `exec.Command` 来执行命令,但是并没有设置 `cmd.Dir` 来指定命令执行的工作目录。如果命令需要在特定的工作目录下执行,您可以设置 `cmd.Dir` 来指定。例如:
```
cmd := exec.Command("ls")
cmd.Dir = "/home/user"
output, err := cmd.CombinedOutput()
if err != nil {
log.Fatal(err)
}
fmt.Println(string(output))
```
另外,执行 Shell 命令时最好使用 `bash` 而不是 `sh`,因为 `sh` 的行为可能会与您预期的不同。如果您的系统上没有 `bash`,可以尝试安装一下。
阅读全文
相关推荐
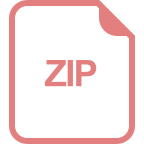
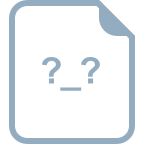
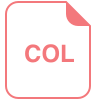
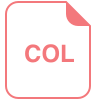
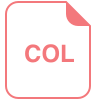
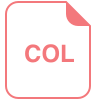
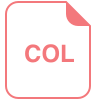
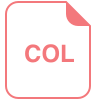
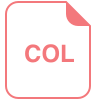
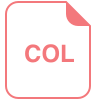
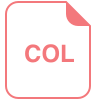
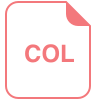
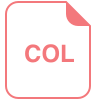
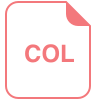

