写一个基于vue2.0的富文本编辑器
时间: 2024-05-13 12:19:00 浏览: 236
好的,我可以给你提供一个基于 vue2.0 的富文本编辑器的实现思路。
首先,我们需要引入一个富文本编辑器的库,比较常用的有Quill、WangEditor、TinyMCE等。这里以Quill为例进行实现。
1. 安装Quill
```
npm install quill --save
```
2. 在Vue组件中引入Quill
```vue
<template>
<div id="editor"></div>
</template>
<script>
import Quill from 'quill'
export default {
mounted() {
this.quillEditor = new Quill('#editor', {
modules: {
toolbar: [
['bold', 'italic', 'underline', 'strike'], // 加粗、斜体、下划线、删除线
['blockquote', 'code-block'], // 引用、代码块
[{ 'header': 1 }, { 'header': 2 }], // 标题
[{ 'list': 'ordered' }, { 'list': 'bullet' }], // 列表
[{ 'script': 'sub' }, { 'script': 'super' }], // 上下标
[{ 'indent': '-1' }, { 'indent': '+1' }], // 缩进
[{ 'direction': 'rtl' }, { 'align': [] }], // 对齐方式
[{ 'color': [] }, { 'background': [] }], // 颜色、背景色
['image', 'video'], // 图片、视频
['clean'] // 清除格式
]
},
placeholder: '请输入内容',
theme: 'snow' // 主题,有两个值可选:snow和bubble
})
}
}
</script>
<style>
/* Quill需要的CSS */
@import "~quill/dist/quill.snow.css";
</style>
```
这样就可以得到一个基本的富文本编辑器了。但是这个富文本编辑器不能与Vue组件的数据进行双向绑定,我们需要对Quill进行一些扩展。
3. 扩展Quill
我们需要在Quill的change事件中,把编辑器中的内容同步到Vue组件的data中。同时,当Vue组件的data中的值发生变化时,要把新的值同步到Quill中。
```vue
<template>
<div id="editor" ref="editor"></div>
</template>
<script>
import Quill from 'quill'
export default {
data() {
return {
content: ''
}
},
mounted() {
this.quillEditor = new Quill(this.$refs.editor, {
modules: {
toolbar: [
['bold', 'italic', 'underline', 'strike'], // 加粗、斜体、下划线、删除线
['blockquote', 'code-block'], // 引用、代码块
[{ 'header': 1 }, { 'header': 2 }], // 标题
[{ 'list': 'ordered' }, { 'list': 'bullet' }], // 列表
[{ 'script': 'sub' }, { 'script': 'super' }], // 上下标
[{ 'indent': '-1' }, { 'indent': '+1' }], // 缩进
[{ 'direction': 'rtl' }, { 'align': [] }], // 对齐方式
[{ 'color': [] }, { 'background': [] }], // 颜色、背景色
['image', 'video'], // 图片、视频
['clean'] // 清除格式
]
},
placeholder: '请输入内容',
theme: 'snow' // 主题,有两个值可选:snow和bubble
})
// 把编辑器中的内容同步到Vue组件的data中
this.quillEditor.on('text-change', () => {
this.content = this.quillEditor.root.innerHTML
})
// 把Vue组件的data中的值同步到Quill中
this.$watch('content', (newValue) => {
this.quillEditor.root.innerHTML = newValue
})
}
}
</script>
<style>
/* Quill需要的CSS */
@import "~quill/dist/quill.snow.css";
</style>
```
现在,我们就可以在Vue组件中使用这个富文本编辑器,并且能够与Vue组件的data进行双向绑定了。
```vue
<template>
<div>
<editor v-model="content"></editor>
<div v-html="content"></div>
</div>
</template>
<script>
import Editor from './Editor.vue'
export default {
components: {
Editor
},
data() {
return {
content: ''
}
}
}
</script>
```
阅读全文
相关推荐
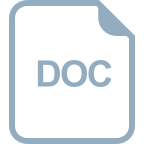
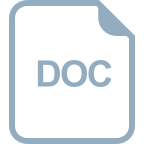
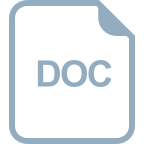
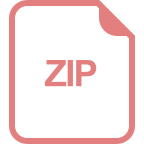
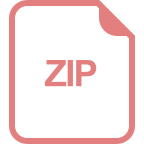
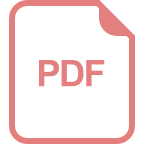

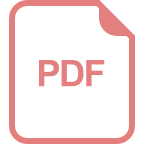
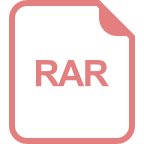
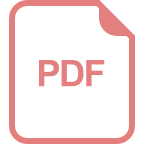
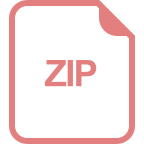
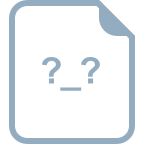
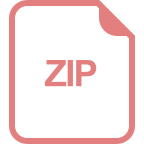
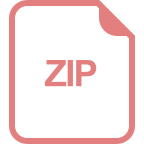
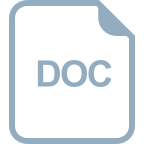

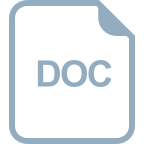
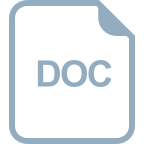