spring boot aop如何使用
时间: 2023-10-30 22:07:22 浏览: 168
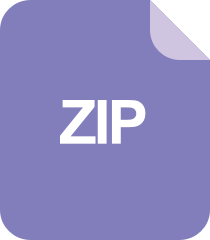
spring-boot-aop.zip
Spring Boot AOP(面向切面编程)是一种技术,可以在应用程序执行期间拦截和修改方法调用。使用AOP,您可以在不修改原始代码的情况下添加功能,例如日志记录、性能监控、安全性等。
以下是使用Spring Boot AOP的步骤:
1. 添加依赖项:在pom.xml文件中添加Spring AOP依赖项。
```
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-aop</artifactId>
</dependency>
```
2. 创建切面类:创建一个类,并使用@Aspect注释标记它。在切面类中,您可以定义一个或多个切点和通知方法。切点表示在应用程序中要拦截的方法,通知是在切点处执行的代码。
```
@Aspect
@Component
public class LoggingAspect {
@Before("execution(* com.example.demo.service.*.*(..))")
public void logBefore(JoinPoint joinPoint) {
System.out.println("Before method execution: " + joinPoint.getSignature().getName());
}
@After("execution(* com.example.demo.service.*.*(..))")
public void logAfter(JoinPoint joinPoint) {
System.out.println("After method execution: " + joinPoint.getSignature().getName());
}
}
```
上面的示例中,我们创建了一个LoggingAspect类,并使用@Component注释将其标记为Spring组件。我们还定义了两个通知方法:logBefore()和logAfter()。这些方法在执行拦截的方法之前和之后执行。
3. 配置AOP:在Spring Boot应用程序的配置文件中,添加以下内容:
```
spring.aop.auto=true
```
此配置将自动启用Spring AOP。
4. 测试AOP:创建一个简单的Spring Boot服务,并在其中调用要拦截的方法。
```
@RestController
@RequestMapping("/api")
public class DemoController {
@Autowired
private DemoService demoService;
@GetMapping("/hello")
public String sayHello() {
return demoService.sayHello();
}
}
```
在上面的示例中,我们创建了一个DemoController类,并使用@RestController和@RequestMapping注释将其标记为Spring MVC控制器。我们还注入了一个DemoService类,并在sayHello()方法中调用它的方法。
在这里,如果我们想要在调用sayHello()方法之前和之后记录日志,我们可以使用LoggingAspect类中定义的通知方法。在执行应用程序时,您应该看到类似以下内容的输出:
```
Before method execution: sayHello
After method execution: sayHello
```
这表明我们已成功使用Spring Boot AOP拦截和修改方法调用。
阅读全文
相关推荐
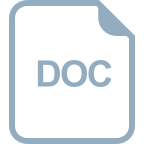
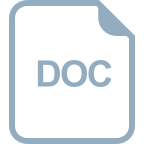
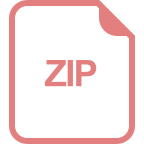
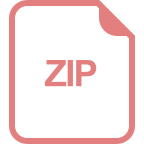

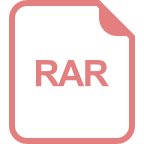
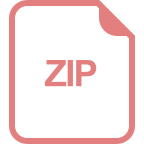
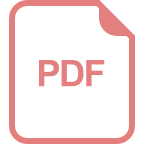
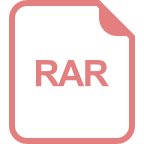
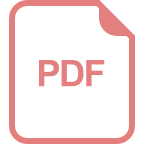
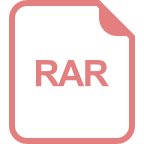
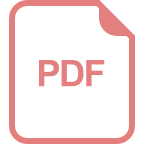
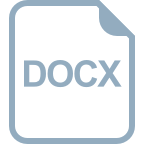
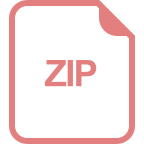
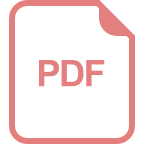
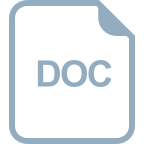
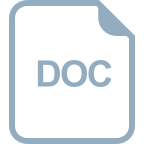