双边滤波算法python怎么写?
时间: 2023-10-08 13:06:53 浏览: 75
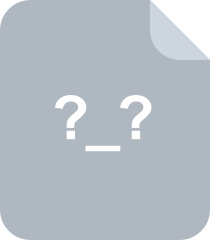
双边滤波算法

双边滤波算法是一种常用的图像滤波方法,可以在保持边缘信息的同时进行噪声抑制。下面是使用Python实现双边滤波算法的代码:
```python
import cv2
import numpy as np
def bilateral_filter(img, d, sigma_color, sigma_space):
# 初始化输出图像
out = np.zeros_like(img)
# 获取图像尺寸
height, width = img.shape[:2]
# 计算空间权重系数
space_coef = np.zeros((height, width))
for i in range(height):
for j in range(width):
space_coef[i, j] = np.exp(-(i ** 2 + j ** 2) / (2 * sigma_space ** 2))
# 双边滤波
for i in range(height):
for j in range(width):
# 确定当前像素的滤波范围
i_min = max(i - d, 0)
i_max = min(i + d, height - 1)
j_min = max(j - d, 0)
j_max = min(j + d, width - 1)
# 计算颜色权重系数
color_coef = np.zeros((i_max - i_min + 1, j_max - j_min + 1))
for k in range(i_min, i_max + 1):
for l in range(j_min, j_max + 1):
color_coef[k - i_min, l - j_min] = np.exp(-((img[k, l] - img[i, j]) ** 2) / (2 * sigma_color ** 2))
# 计算双边滤波系数
coef = color_coef * space_coef[i_min:i_max + 1, j_min:j_max + 1]
coef_sum = np.sum(coef)
# 计算输出像素值
out[i, j] = np.sum(coef * img[i_min:i_max + 1, j_min:j_max + 1]) / coef_sum
return out
# 读取图像
img = cv2.imread('lena.png', 0)
# 双边滤波
out = bilateral_filter(img, 5, 50, 50)
# 显示结果
cv2.imshow('Original', img)
cv2.imshow('Bilateral Filter', out)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
在上述代码中,bilateral_filter()函数接受四个参数:输入图像img、滤波范围d、颜色空间标准差sigma_color和空间标准差sigma_space。函数首先初始化输出图像out,然后计算空间权重系数space_coef。接下来,对于输入图像中的每个像素,确定其滤波范围,计算颜色权重系数color_coef,并将其与空间权重系数相乘得到双边滤波系数coef。通过对coef进行归一化,最终计算输出像素值out[i, j]。最后,通过调用cv2.imshow()函数显示原始图像和双边滤波结果。
阅读全文
相关推荐



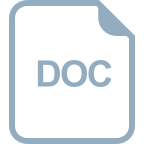
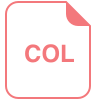





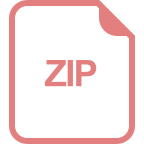
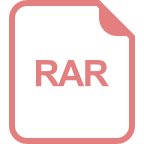



