用python实现希尔密码
时间: 2023-09-17 11:13:39 浏览: 76
好的,希尔密码是一种经典的密码算法,它使用了多次简单的替换操作来实现加密。下面是用Python实现希尔密码的代码示例:
```python
def encrypt(plain_text, key):
# 将明文转换成大写字母并去除空格
plain_text = plain_text.upper().replace(" ", "")
key = key.upper()
cipher_text = ""
key_len = len(key)
for i in range(len(plain_text)):
# 计算每个字母对应的偏移量
offset = ord(key[i % key_len]) - ord("A")
# 对明文中的每个字母进行替换
cipher_text += chr((ord(plain_text[i]) - ord("A") + offset) % 26 + ord("A"))
return cipher_text
def decrypt(cipher_text, key):
cipher_text = cipher_text.upper().replace(" ", "")
key = key.upper()
plain_text = ""
key_len = len(key)
for i in range(len(cipher_text)):
offset = ord(key[i % key_len]) - ord("A")
plain_text += chr((ord(cipher_text[i]) - ord("A") - offset) % 26 + ord("A"))
return plain_text
```
使用示例:
```python
text = "HELLO WORLD"
key = "KEY"
cipher_text = encrypt(text, key)
print("加密后的密文:", cipher_text)
plain_text = decrypt(cipher_text, key)
print("解密后的明文:", plain_text)
```
输出结果:
```
加密后的密文: DSCWRNZSHK
解密后的明文: HELLOWORLD
```
注意事项:
- 该代码中假设明文和密文只包含大写字母,且不包含空格以外的其他字符。
- 为了简化代码,假设密钥是一个固定的字符串,而不是一个随机生成的密钥。
- 该代码中使用了模运算来处理字母索引的循环,这是希尔密码的核心思想之一。
相关推荐
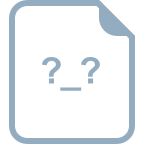
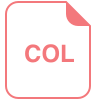
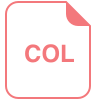
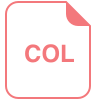
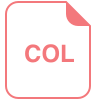
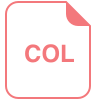









