python给我的塔防游戏地图设置起点和终点
时间: 2023-11-16 16:31:28 浏览: 111
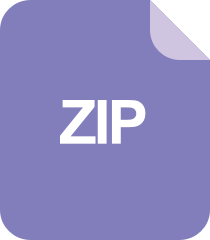
Python塔防游戏
为了给塔防游戏地图设置起点和终点,你可以使用 Python 中的 Pygame 模块来实现。具体的步骤如下:
1. 在 Pygame 中加载地图图片,并使用鼠标或触摸屏选择起点和终点的位置,可以使用 Pygame 提供的事件监听功能来实现。
```python
import pygame
# 初始化 Pygame
pygame.init()
# 加载地图图片
map_image = pygame.image.load("map.png")
# 创建 Pygame 窗口
screen = pygame.display.set_mode((map_image.get_width(), map_image.get_height()))
# 循环监听事件
while True:
for event in pygame.event.get():
if event.type == pygame.MOUSEBUTTONUP:
# 获取鼠标点击的位置
mouse_x, mouse_y = pygame.mouse.get_pos()
# 判断点击的位置是起点还是终点
if is_start_position(mouse_x, mouse_y):
set_start_position(mouse_x, mouse_y)
elif is_end_position(mouse_x, mouse_y):
set_end_position(mouse_x, mouse_y)
elif event.type == pygame.QUIT:
pygame.quit()
sys.exit()
# 在窗口中显示地图图片
screen.blit(map_image, (0, 0))
# 在窗口中显示起点和终点
draw_start_position()
draw_end_position()
# 更新窗口显示
pygame.display.update()
```
2. 在 Pygame 中保存起点和终点的位置信息,可以使用 Python 的字典或列表来存储。
```python
# 定义起点和终点的位置信息
start_position = None
end_position = None
# 存储起点和终点的位置信息
position_dict = {
"start": start_position,
"end": end_position
}
# 设置起点和终点的位置信息
def set_start_position(x, y):
position_dict["start"] = (x, y)
def set_end_position(x, y):
position_dict["end"] = (x, y)
# 判断一个点是否是起点或终点
def is_start_position(x, y):
if start_position and abs(x - start_position[0]) <= 10 and abs(y - start_position[1]) <= 10:
return True
else:
return False
def is_end_position(x, y):
if end_position and abs(x - end_position[0]) <= 10 and abs(y - end_position[1]) <= 10:
return True
else:
return False
# 在窗口中显示起点和终点
def draw_start_position():
if start_position:
pygame.draw.circle(screen, (255, 0, 0), start_position, 10)
def draw_end_position():
if end_position:
pygame.draw.circle(screen, (0, 255, 0), end_position, 10)
```
3. 在 Pygame 中计算起点和终点之间的最短路径或最优路径,可以使用 Python 的路径规划库,例如 NetworkX 或 A* 算法库。
```python
import networkx as nx
# 创建地图的图形表示
map_graph = nx.Graph()
# 添加地图的节点和边
# ...
# 计算起点和终点之间的最短路径
if start_position and end_position:
start_node = get_closest_node(start_position, map_graph)
end_node = get_closest_node(end_position, map_graph)
shortest_path = nx.shortest_path(map_graph, start_node, end_node)
# 绘制最短路径
if shortest_path:
pygame.draw.lines(screen, (0, 0, 255), False, shortest_path, 5)
```
需要注意的是,具体的实现方式可能会因为使用的地图编辑器或开发工具包而有所不同。
阅读全文
相关推荐
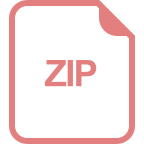
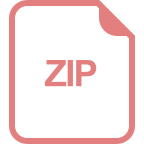
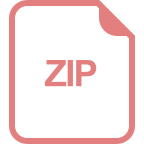
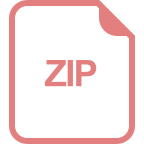
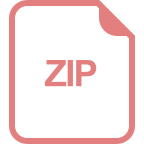
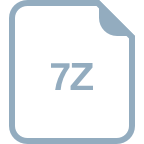
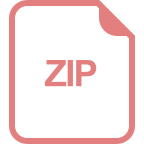
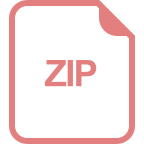
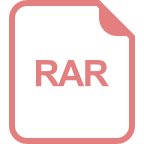
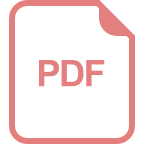
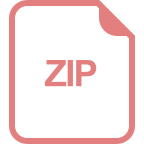
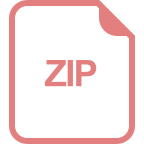
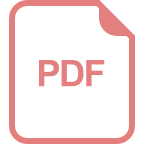
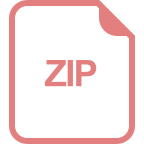