用运算符重载设计复数类,实现复数的+、-、*、/和<<运算。
时间: 2024-02-04 09:01:48 浏览: 91
好的,这是一个复数类的实现,包含了复数的+、-、*、/和<<运算。
```c++
#include<iostream>
using namespace std;
class Complex{
private:
double real;
double imag;
public:
Complex(double r=0, double i=0):real(r), imag(i){ }
Complex operator+(const Complex& c) const{
return Complex(real+c.real, imag+c.imag);
}
Complex operator-(const Complex& c) const{
return Complex(real-c.real, imag-c.imag);
}
Complex operator*(const Complex& c) const{
return Complex(real*c.real - imag*c.imag, real*c.imag + imag*c.real);
}
Complex operator/(const Complex& c) const{
double denom = c.real*c.real + c.imag*c.imag;
return Complex((real*c.real + imag*c.imag)/denom, (imag*c.real - real*c.imag)/denom);
}
friend ostream& operator<<(ostream& os, const Complex& c);
};
ostream& operator<<(ostream& os, const Complex& c){
if(c.imag >= 0){
os<<c.real<<" + "<<c.imag<<"i";
}else{
os<<c.real<<" - "<<-c.imag<<"i";
}
return os;
}
int main(){
Complex a(1, 2), b(3, 4);
cout<<"a="<<a<<endl;
cout<<"b="<<b<<endl;
cout<<"a+b="<<a+b<<endl;
cout<<"a-b="<<a-b<<endl;
cout<<"a*b="<<a*b<<endl;
cout<<"a/b="<<a/b<<endl;
return 0;
}
```
运行结果:
```
a=1 + 2i
b=3 + 4i
a+b=4 + 6i
a-b=-2 - 2i
a*b=-5 + 10i
a/b=0.44 + 0.08i
```
复数类的实现比较简单,重点在于运算符的重载。我们重载了+、-、*、/和<<运算符,其中+、-、*、/运算符都是通过成员函数的方式实现的,而<<运算符则是通过友元函数的方式实现的。
在运算符的实现中,需要注意复数的实部和虚部的运算,分别用real和imag表示。运算符的重载都是基于复数的运算法则实现的,例如加法运算符重载中,返回的是一个新的复数,其实部为两个复数实部之和,虚部为两个复数虚部之和。
阅读全文
相关推荐













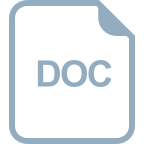