QT实现一个智能系统代码
时间: 2024-05-03 22:18:11 浏览: 26
要实现一个智能系统,首先需要明确系统的功能和任务。一般而言,智能系统需要具备自然语言处理、机器学习、知识图谱等技术。
下面是一个基于QT的简单智能系统示例,实现了一个简单的聊天机器人。
```c++
#include <QGuiApplication>
#include <QQmlApplicationEngine>
#include <QObject>
#include <QString>
#include <QVariant>
#include "chatbot.h"
int main(int argc, char *argv[])
{
QGuiApplication app(argc, argv);
// Create the chat bot
ChatBot bot;
// Expose the chat bot to QML
QQmlApplicationEngine engine;
engine.rootContext()->setContextProperty("chatBot", &bot);
engine.load(QUrl(QStringLiteral("qrc:/main.qml")));
return app.exec();
}
```
在这个示例中,我们创建了一个名为ChatBot的类,用于实现聊天机器人的功能。然后,我们将这个类实例化并将其暴露给QML界面。
```c++
class ChatBot : public QObject
{
Q_OBJECT
public:
ChatBot(QObject *parent = nullptr);
public slots:
QVariantList getResponse(const QString &message);
private:
// Some private member variables and functions
};
```
ChatBot类中,我们定义了一个getResponse函数,用于接收用户的消息并返回机器人的回复。这个函数可以在QML界面中被调用。
```c++
QVariantList ChatBot::getResponse(const QString &message)
{
// Process the message and generate a response
//
QVariantList responseList;
responseList.append("Bot: " + response);
return responseList;
}
```
在getResponse函数中,我们使用自然语言处理技术对用户输入的消息进行处理,并生成一个回复消息。最后,将回复消息以QVariantList的形式返回给QML界面。在QML界面中,我们可以使用JavaScript调用getResponse函数并显示机器人的回复。
```qml
import QtQuick 2.0
import QtQuick.Controls 2.12
ApplicationWindow {
visible: true
width: 640
height: 480
title: "Chat Bot"
Column {
spacing: 5
anchors.centerIn: parent
TextField {
id: messageField
placeholderText: "Enter your message"
}
Button {
text: "Send"
onClicked: {
var message = messageField.text;
messageField.text = "";
var responseList = chatBot.getResponse(message);
for (var i = 0; i < responseList.length; ++i) {
chatLog.append(responseList[i]);
}
}
}
ListView {
id: chatLog
width: parent.width
height: parent.height - messageField.height - sendButton.height
model: ListModel {
}
delegate: Text {
text: modelData
font.pointSize: 16
}
}
}
}
```
在QML界面中,我们使用TextField获取用户输入的消息,并在Button的点击事件中调用ChatBot类的getResponse函数,将机器人的回复添加到ListView中显示给用户。
当然,这只是一个简单的示例。要实现更加复杂的智能系统,需要使用更多的技术和算法,并进行更加细致的设计和实现。
相关推荐
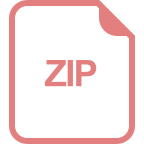










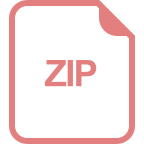
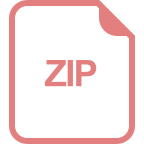
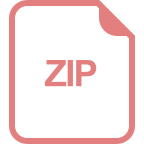