教务系统springboot代码实现
时间: 2023-06-16 09:04:35 浏览: 75
教务系统是一个大型的信息系统,包括多个模块,如学生管理、教师管理、课程管理、成绩管理等等。这里给出一个简单的教务系统学生管理模块的Spring Boot代码实现。
1. 创建一个Spring Boot项目
在Eclipse或者IntelliJ IDEA中创建一个Spring Boot项目,选择Web依赖,生成一个基本的Spring Boot工程。
2. 添加相关依赖
在项目中添加一些必要的依赖,如JPA、MySQL、Thymeleaf等。在pom.xml中添加以下依赖:
```xml
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
</dependency>
</dependencies>
```
3. 配置数据库连接
在application.properties中添加数据库连接信息:
```properties
spring.datasource.url=jdbc:mysql://localhost:3306/edu_system?useSSL=false
spring.datasource.username=root
spring.datasource.password=root
spring.datasource.driver-class-name=com.mysql.jdbc.Driver
spring.jpa.database-platform=org.hibernate.dialect.MySQL5Dialect
spring.jpa.hibernate.ddl-auto=update
```
其中,edu_system是数据库名称,root是数据库用户名和密码。
4. 创建实体类
创建一个学生实体类,包括学生编号、学生姓名、学生性别、学生年龄等属性。代码实现如下:
```java
@Entity
@Table(name="student")
public class Student {
@Id
@GeneratedValue(strategy=GenerationType.AUTO)
private Long id;
@Column(name="student_no")
private String studentNo;
@Column(name="student_name")
private String studentName;
@Column(name="student_sex")
private String studentSex;
@Column(name="student_age")
private int studentAge;
//省略getter和setter方法
}
```
5. 创建数据访问层
创建一个学生数据访问层接口,继承JpaRepository,用于对学生数据进行CRUD操作。代码实现如下:
```java
@Repository
public interface StudentRepository extends JpaRepository<Student, Long> {
}
```
6. 创建服务层
创建一个学生服务层接口,用于对学生数据进行业务逻辑处理。代码实现如下:
```java
public interface StudentService {
public void add(Student student);
public void delete(Long id);
public void update(Student student);
public Student findById(Long id);
public List<Student> findAll();
}
```
创建一个学生服务层实现类,实现StudentService接口,调用数据访问层接口的方法对学生数据进行操作。代码实现如下:
```java
@Service
public class StudentServiceImpl implements StudentService {
@Autowired
private StudentRepository studentRepository;
@Override
public void add(Student student) {
studentRepository.save(student);
}
@Override
public void delete(Long id) {
studentRepository.deleteById(id);
}
@Override
public void update(Student student) {
studentRepository.save(student);
}
@Override
public Student findById(Long id) {
return studentRepository.findById(id).get();
}
@Override
public List<Student> findAll() {
return studentRepository.findAll();
}
}
```
7. 创建控制器
创建一个学生控制器,用于接受用户的请求,调用服务层接口的方法处理请求,并返回视图。代码实现如下:
```java
@Controller
@RequestMapping("/student")
public class StudentController {
@Autowired
private StudentService studentService;
@GetMapping("/list")
public String list(Model model) {
List<Student> students = studentService.findAll();
model.addAttribute("students", students);
return "student/list";
}
@GetMapping("/add")
public String add(Model model) {
Student student = new Student();
model.addAttribute("student", student);
return "student/add";
}
@PostMapping("/add")
public String add(@ModelAttribute("student") Student student) {
studentService.add(student);
return "redirect:/student/list";
}
@GetMapping("/delete/{id}")
public String delete(@PathVariable("id") Long id) {
studentService.delete(id);
return "redirect:/student/list";
}
@GetMapping("/update/{id}")
public String update(@PathVariable("id") Long id, Model model) {
Student student = studentService.findById(id);
model.addAttribute("student", student);
return "student/update";
}
@PostMapping("/update")
public String update(@ModelAttribute("student") Student student) {
studentService.update(student);
return "redirect:/student/list";
}
}
```
8. 创建视图
在resources/templates目录下创建学生列表、学生添加、学生修改的HTML视图文件。
(1)学生列表视图(list.html):
```html
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>学生列表</title>
</head>
<body>
<h1>学生列表</h1>
<table border="1">
<tr>
<th>编号</th>
<th>学号</th>
<th>姓名</th>
<th>性别</th>
<th>年龄</th>
<th>操作</th>
</tr>
<tr th:each="student : ${students}">
<td th:text="${student.id}"></td>
<td th:text="${student.studentNo}"></td>
<td th:text="${student.studentName}"></td>
<td th:text="${student.studentSex}"></td>
<td th:text="${student.studentAge}"></td>
<td>
<a th:href="@{/student/update/{id}(id=${student.id})}">修改</a>
<a th:href="@{/student/delete/{id}(id=${student.id})}">删除</a>
</td>
</tr>
</table>
<a href="/student/add">添加学生</a>
</body>
</html>
```
(2)学生添加视图(add.html):
```html
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>添加学生</title>
</head>
<body>
<h1>添加学生</h1>
<form action="/student/add" method="post">
<table>
<tr>
<td>学号:</td>
<td><input type="text" name="studentNo" th:value="${student.studentNo}" /></td>
</tr>
<tr>
<td>姓名:</td>
<td><input type="text" name="studentName" th:value="${student.studentName}" /></td>
</tr>
<tr>
<td>性别:</td>
<td><input type="text" name="studentSex" th:value="${student.studentSex}" /></td>
</tr>
<tr>
<td>年龄:</td>
<td><input type="text" name="studentAge" th:value="${student.studentAge}" /></td>
</tr>
<tr>
<td colspan="2"><input type="submit" value="添加" /></td>
</tr>
</table>
</form>
<a href="/student/list">返回列表</a>
</body>
</html>
```
(3)学生修改视图(update.html):
```html
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>修改学生</title>
</head>
<body>
<h1>修改学生</h1>
<form action="/student/update" method="post">
<input type="hidden" name="id" th:value="${student.id}" />
<table>
<tr>
<td>学号:</td>
<td><input type="text" name="studentNo" th:value="${student.studentNo}" /></td>
</tr>
<tr>
<td>姓名:</td>
<td><input type="text" name="studentName" th:value="${student.studentName}" /></td>
</tr>
<tr>
<td>性别:</td>
<td><input type="text" name="studentSex" th:value="${student.studentSex}" /></td>
</tr>
<tr>
<td>年龄:</td>
<td><input type="text" name="studentAge" th:value="${student.studentAge}" /></td>
</tr>
<tr>
<td colspan="2"><input type="submit" value="修改" /></td>
</tr>
</table>
</form>
<a href="/student/list">返回列表</a>
</body>
</html>
```
9. 运行测试
启动Spring Boot项目,在浏览器中访问http://localhost:8080/student/list,可以看到学生列表。点击“添加学生”按钮,进入学生添加页面,填写学生信息并提交表单,学生信息被保存到数据库中。点击“修改”按钮,进入学生修改页面,修改学生信息并提交表单,学生信息被更新到数据库中。点击“删除”按钮,学生信息被从数据库中删除。
相关推荐
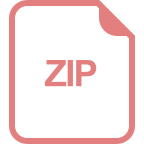
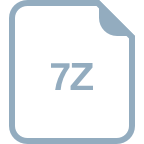














