如何使用python在图片的频域数据做一些处理用于纠正歪斜的图片
时间: 2024-05-01 21:24:03 浏览: 79
纠正歪斜的图片可以使用傅里叶变换来实现。具体步骤如下:
1. 将图片转换为灰度图像。
2. 对灰度图像进行傅里叶变换,得到频域数据。
3. 计算频域数据的幅度谱和相位谱。
4. 计算幅度谱的中心点坐标,并将幅度谱沿中心点旋转相应角度,同时将相位谱也进行同样的旋转。
5. 对旋转后的幅度谱和相位谱进行逆傅里叶变换,得到纠正后的图像。
下面是使用Python实现纠正歪斜的图片的示例代码:
```python
import cv2
import numpy as np
def correct_skewed_image(image):
# Convert image to grayscale
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# Apply Fourier transform
f = np.fft.fft2(gray)
# Shift zero frequency component to center of spectrum
fshift = np.fft.fftshift(f)
# Compute magnitude spectrum
magnitude_spectrum = 20*np.log(np.abs(fshift))
# Compute phase spectrum
phase_spectrum = np.angle(fshift)
# Find center of magnitude spectrum
rows, cols = gray.shape
crow, ccol = int(rows/2), int(cols/2)
# Compute gradient of magnitude spectrum
magnitude_spectrum_grad = cv2.Sobel(magnitude_spectrum, cv2.CV_64F, 1, 1, ksize=5)
# Compute angle of maximum gradient
_, _, _, max_loc = cv2.minMaxLoc(magnitude_spectrum_grad)
angle = np.arctan2(max_loc[1] - crow, max_loc[0] - ccol) * 180 / np.pi
# Rotate magnitude and phase spectra
rotated_magnitude_spectrum = np.rot90(magnitude_spectrum, -1)
rotated_phase_spectrum = np.rot90(phase_spectrum, -1)
# Shift spectra back to original position
rotated_magnitude_spectrum = np.fft.ifftshift(rotated_magnitude_spectrum)
rotated_phase_spectrum = np.fft.ifftshift(rotated_phase_spectrum)
# Compute inverse Fourier transform
f_ = np.real(np.fft.ifft2(np.exp(rotated_magnitude_spectrum + 1j*rotated_phase_spectrum)))
# Convert back to uint8
f_ = np.uint8(f_)
return f_
```
在上面的代码中,我们首先将图像转换为灰度图像,然后对灰度图像进行傅里叶变换,并计算出幅度和相位谱。然后,我们找到幅度谱的中心点坐标,并计算出幅度谱的梯度,从而可以找到梯度最大的方向,即图像的旋转角度。接着,我们将幅度谱和相位谱旋转相应角度,并进行逆傅里叶变换,得到纠正后的图像。
使用以上代码,你可以通过调用`correct_skewed_image`函数来对图片进行纠正,例如:
```python
image = cv2.imread('skewed_image.png')
corrected_image = correct_skewed_image(image)
cv2.imwrite('corrected_image.png', corrected_image)
```
阅读全文
相关推荐
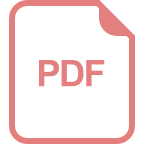






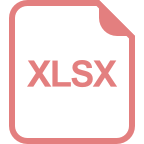
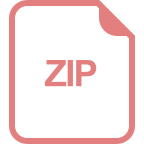
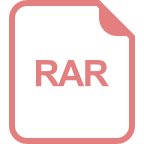
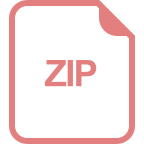
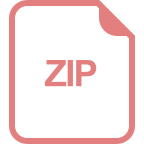
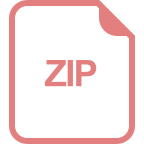
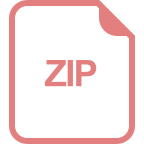
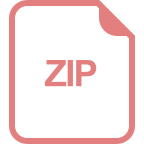
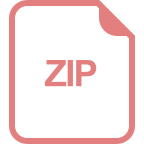
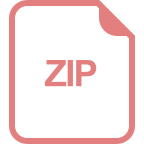
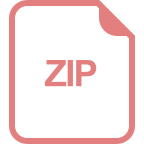