写一个基于Python与tkinter名片识别的代码
时间: 2024-03-23 15:39:40 浏览: 75
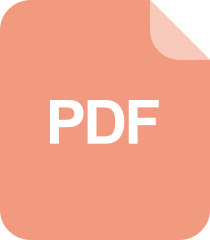
基于python tkinter的点名小程序功能的实例代码
以下是一个基于Python和tkinter的名片识别代码:
```python
import tkinter as tk
import cv2
import numpy as np
import pytesseract
from PIL import ImageTk, Image
pytesseract.pytesseract.tesseract_cmd = r"C:\Program Files\Tesseract-OCR\tesseract.exe" # 指定 tesseract OCR 的安装路径
class App:
def __init__(self, window, window_title):
self.window = window
self.window.title(window_title)
# 创建一个标签,显示摄像头捕获的图像
self.image_label = tk.Label(self.window)
self.image_label.pack()
# 创建一个按钮,用于开始名片识别
self.btn_snapshot = tk.Button(self.window, text="开始名片识别", width=50, command=self.snapshot)
self.btn_snapshot.pack(anchor=tk.CENTER, expand=True)
self.video_capture = cv2.VideoCapture(0) # 打开摄像头
self.delay = 15 # 延迟15毫秒更新图像
self.update() # 启动更新图像的方法
self.window.mainloop()
def snapshot(self):
# 获取当前帧的图像
ret, frame = self.video_capture.read()
# 调整图像大小
frame = cv2.resize(frame, (640,480))
# 对图像进行处理,提取名片上的文字
gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
gray = cv2.threshold(gray, 0, 255, cv2.THRESH_BINARY | cv2.THRESH_OTSU)[1]
gray = cv2.medianBlur(gray, 3)
filename = "temp.png"
cv2.imwrite(filename, gray)
text = pytesseract.image_to_string(Image.open(filename))
# 显示处理后的图像和识别出的文字
cv2.imshow("Processed Image", gray)
print(text)
def update(self):
ret, frame = self.video_capture.read()
if ret:
# 调整图像大小
frame = cv2.resize(frame, (640,480))
# 在图像上绘制一个矩形,用于框定名片区域
cv2.rectangle(frame, (200,100), (440,380), (0,255,0), 2)
# 将 OpenCV 图像转换为 tkinter 图像
image = cv2.cvtColor(frame, cv2.COLOR_BGR2RGB)
image = Image.fromarray(image)
image = ImageTk.PhotoImage(image)
# 显示 tkinter 图像
self.image_label.configure(image=image)
self.image_label.image = image
self.window.after(self.delay, self.update)
# 启动应用程序
App(tk.Tk(), "名片识别")
```
这个程序使用 OpenCV 捕获摄像头的图像,并使用 pytesseract 库提取名片上的文字。在 tkinter 界面中,用户可以点击“开始名片识别”按钮开始识别名片。程序会在摄像头捕获的图像中,框定名片区域,然后提取该区域上的文字,并在控制台输出识别结果。
阅读全文
相关推荐

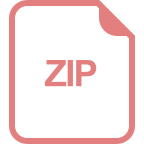
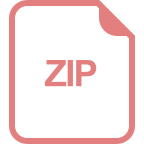
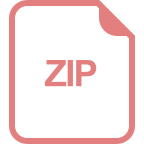
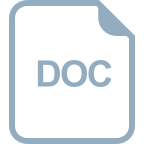
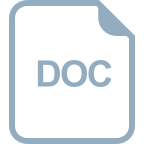



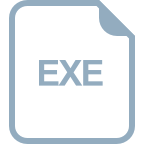