stm32f103c8t6,串口1;一帧数据=帧头+数据+帧尾;帧头为FF,数据范围为00-3F,帧尾EE;接收数据为FF开始接收,接收为EE接收结束;一帧数据接收结束后,提取数据传值给last_zero_count;
时间: 2024-05-03 16:22:23 浏览: 75
以下是基于STM32F103C8T6的串口1接收数据并提取数据的示例代码:
```
#include "stm32f10x.h"
#define FRAME_HEADER 0xFF
#define FRAME_TAIL 0xEE
uint8_t last_zero_count = 0; // 存放提取的数据
void USART1_IRQHandler(void)
{
if (USART_GetITStatus(USART1, USART_IT_RXNE) != RESET) // 判断是否接收到数据
{
static uint8_t rx_buffer[3]; // 缓存数组,存储一帧数据的帧头、数据、帧尾
static uint8_t rx_index = 0; // 缓存数组的索引
uint16_t data = USART_ReceiveData(USART1) & 0xFF; // 读取接收到的数据
if (rx_index == 0 && data == FRAME_HEADER) // 如果读取到帧头
{
rx_buffer[0] = data; // 存储帧头
rx_index++; // 缓存索引加1
}
else if (rx_index == 1 && (data >= 0x00 && data <= 0x3F)) // 如果读取到数据
{
rx_buffer[1] = data; // 存储数据
rx_index++; // 缓存索引加1
}
else if (rx_index == 2 && data == FRAME_TAIL) // 如果读取到帧尾
{
rx_buffer[2] = data; // 存储帧尾
last_zero_count = rx_buffer[1]; // 提取数据,传值给last_zero_count
rx_index = 0; // 缓存索引清零,准备接收下一帧数据
}
else // 如果读取到的数据不符合一帧数据的格式,或者缓存数组已满
{
rx_index = 0; // 缓存索引清零,准备接收下一帧数据
}
}
}
int main(void)
{
// 初始化串口1
USART_InitTypeDef USART_InitStruct;
GPIO_InitTypeDef GPIO_InitStruct;
NVIC_InitTypeDef NVIC_InitStruct;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_USART1 | RCC_APB2Periph_GPIOA, ENABLE);
GPIO_InitStruct.GPIO_Pin = GPIO_Pin_9;
GPIO_InitStruct.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_Init(GPIOA, &GPIO_InitStruct);
GPIO_InitStruct.GPIO_Pin = GPIO_Pin_10;
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_Init(GPIOA, &GPIO_InitStruct);
USART_InitStruct.USART_BaudRate = 9600;
USART_InitStruct.USART_WordLength = USART_WordLength_8b;
USART_InitStruct.USART_StopBits = USART_StopBits_1;
USART_InitStruct.USART_Parity = USART_Parity_No;
USART_InitStruct.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStruct.USART_Mode = USART_Mode_Rx | USART_Mode_Tx;
USART_Init(USART1, &USART_InitStruct);
USART_ITConfig(USART1, USART_IT_RXNE, ENABLE);
NVIC_InitStruct.NVIC_IRQChannel = USART1_IRQn;
NVIC_InitStruct.NVIC_IRQChannelPreemptionPriority = 0;
NVIC_InitStruct.NVIC_IRQChannelSubPriority = 0;
NVIC_InitStruct.NVIC_IRQChannelCmd = ENABLE;
NVIC_Init(&NVIC_InitStruct);
USART_Cmd(USART1, ENABLE);
while (1)
{
// 主程序代码
}
}
```
阅读全文
相关推荐
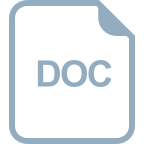
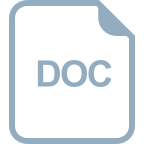
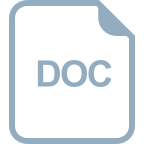
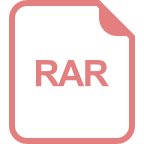
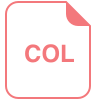
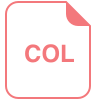




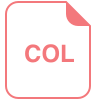
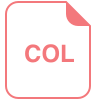
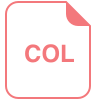

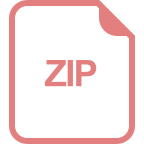
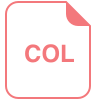
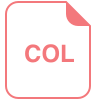
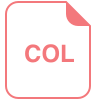
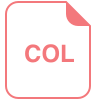
