struct Linke { float data; struct Link *next; }; struct Link * Fun(int n) { struct Link *head =NULL,*p,*pr; int i; for(i =1;i <= n;i++) {p=(struct Link *)malloc(sizeof(struct Link)); p->data = i;e p->next=NULL; if(head -NULL) { head=p; pr=p; } else{ pr->next-p; pr=p; } return head;e }
时间: 2023-05-18 08:04:33 浏览: 54
这是一个定义了一个结构体Link的函数Fun,函数的功能是创建一个链表,链表的结构体Link中有两个成员:一个浮点类型的数据data和一个指向另一个结构体Link的指针next。函数的参数是一个整数n,函数在循环中创建n个Link结构体,并将它们连接成一个链表。 最后,函数返回指向链表开头的指针head。
相关问题
struct node *head=NULL,*tail=NULL,p=NULL;
This code is not valid in C.
The "*" symbol is not used correctly.
Here is a corrected version:
```
struct node *head=NULL,*tail=NULL,*p=NULL;
```
This code declares three pointers to a struct node: head, tail, and p. All three pointers are initialized to NULL.
typedef struct node { int data; struct node *next; } NodeType;
This is a definition of a struct called "node" that has two members: an integer data member called "data" and a pointer to another node struct called "next". The struct is typedef'd as "NodeType" for convenience. This struct can be used to create linked lists, where each node contains a piece of data and a pointer to the next node in the list.
相关推荐
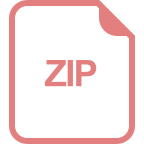
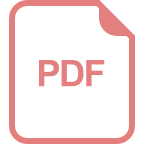













