el-select和el-tree树形结构下拉单选
时间: 2023-10-02 18:14:11 浏览: 141
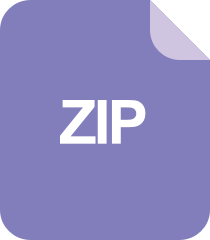
el-tree-select:基于el-select和el-tree改造的树形选择器(下拉框)组件
可以使用 `el-select` 和 `el-tree` 配合使用来实现树形结构下拉单选。
首先,需要将数据转换为 `el-tree` 的节点格式,例如以下数据:
```javascript
const treeData = [
{
id: 1,
label: '节点1',
children: [
{
id: 2,
label: '节点1-1'
},
{
id: 3,
label: '节点1-2'
}
]
},
{
id: 4,
label: '节点2',
children: [
{
id: 5,
label: '节点2-1'
},
{
id: 6,
label: '节点2-2'
}
]
}
]
```
需要转换为 `el-tree` 的节点格式:
```javascript
const treeNodes = [
{
id: 1,
label: '节点1',
children: [
{
id: 2,
label: '节点1-1',
isLeaf: true
},
{
id: 3,
label: '节点1-2',
isLeaf: true
}
]
},
{
id: 4,
label: '节点2',
children: [
{
id: 5,
label: '节点2-1',
isLeaf: true
},
{
id: 6,
label: '节点2-2',
isLeaf: true
}
]
}
]
```
其中,每个节点需要添加 `isLeaf` 属性,用于标记该节点是否为叶子节点。
接着,在 `el-select` 中使用 `el-tree` 作为下拉选项。代码示例:
```html
<template>
<el-select v-model="selectedItem" placeholder="请选择" clearable>
<el-tree :data="treeNodes" :props="treeProps" :node-key="treeProps.id" :highlight-current="true" :default-expand-all="true" :expand-on-click-node="false" v-if="treeVisible"></el-tree>
<el-option v-else v-for="item in options" :key="item.value" :label="item.label" :value="item.value"></el-option>
</el-select>
</template>
<script>
export default {
data() {
return {
selectedItem: null,
treeNodes: [
{
id: 1,
label: '节点1',
children: [
{
id: 2,
label: '节点1-1',
isLeaf: true
},
{
id: 3,
label: '节点1-2',
isLeaf: true
}
]
},
{
id: 4,
label: '节点2',
children: [
{
id: 5,
label: '节点2-1',
isLeaf: true
},
{
id: 6,
label: '节点2-2',
isLeaf: true
}
]
}
],
treeProps: {
children: 'children',
label: 'label',
isLeaf: 'isLeaf'
},
options: [
{
label: '选项1',
value: '1'
},
{
label: '选项2',
value: '2'
},
{
label: '选项3',
value: '3'
}
],
treeVisible: false
}
},
watch: {
selectedItem(val) {
if (val && val.children && val.children.length > 0) {
this.treeVisible = true
} else {
this.treeVisible = false
}
}
}
}
</script>
```
上述代码中,`el-select` 的下拉选项分为两部分:`el-tree` 和 `el-option`。根据当前选中的节点是否有子节点,判断显示 `el-tree` 还是 `el-option`。
在 `el-tree` 中,需要设置 `props` 属性,指定节点数据中的属性名,以及 `node-key` 属性,用于标识节点的唯一属性名。其他属性根据实际需求进行设置。
在 `watch` 中,根据当前选中的节点是否有子节点,控制 `el-tree` 的显示与隐藏。
注意:以上代码只实现了树形结构下拉单选的基本功能,还需要根据实际需求进行修改和完善。
阅读全文
相关推荐
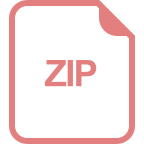
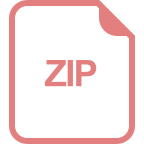
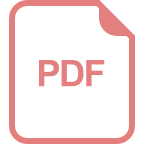





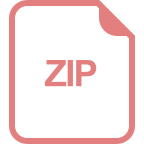
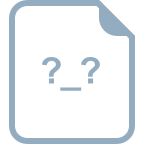
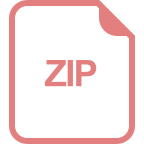
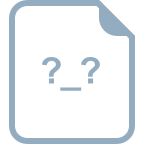
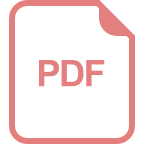
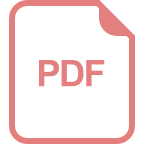
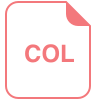

