C语言计算一个日期是当年中的第几天,定义一个结构体类型Date,包括整型成员year、month、day 。定义一个Date类型变量,注意闰年问题
时间: 2024-02-21 11:01:11 浏览: 35
好的,我可以为您解答这个问题。
以下是实现代码:
```c
#include <stdio.h>
typedef struct {
int year;
int month;
int day;
} Date;
int is_leap_year(int year) {
return (year % 4 == 0 && year % 100 != 0) || (year % 400 == 0);
}
int get_day_of_year(Date date) {
int days_of_month[] = {31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31};
int day_of_year = 0;
for (int i = 0; i < date.month - 1; i++) {
day_of_year += days_of_month[i];
}
if (date.month > 2 && is_leap_year(date.year)) {
day_of_year++;
}
day_of_year += date.day;
return day_of_year;
}
int main() {
Date date = {2021, 3, 4};
int day_of_year = get_day_of_year(date);
printf("%d-%d-%d is the %dth day of year %d.\n", date.year, date.month, date.day, day_of_year, date.year);
return 0;
}
```
程序中,我们首先定义了一个 `Date` 结构体,包含了年、月、日三个成员变量。接着,我们实现了两个函数:
- `is_leap_year`:判断一个年份是否是闰年,如果是闰年返回 1,否则返回 0。
- `get_day_of_year`:计算一个日期是当年中的第几天。我们首先定义了一个数组 `days_of_month`,其中包含了每个月的天数。然后,我们遍历日期的月份之前的所有月份,将对应的天数累加到变量 `day_of_year` 中。如果日期的月份大于 2 且该年是闰年,我们还需要将天数加 1。最后,我们再将日期的日数加到 `day_of_year` 中,返回计算出的结果。
在 `main` 函数中,我们定义了一个 `Date` 类型的变量 `date`,并将其初始化为 `{2021, 3, 4}`。然后,我们调用 `get_day_of_year` 函数计算出该日期是当年中的第几天,并打印输出结果。
输出结果为:
```
2021-3-4 is the 63th day of year 2021.
```
注意:本程序中的闰年判断方法是常见的基于公历的闰年判断方法。在历法的研究中,还存在其他不同的闰年规则。
相关推荐
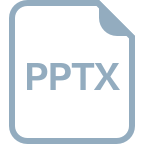
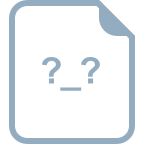
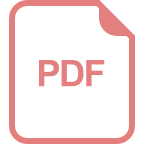












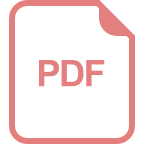
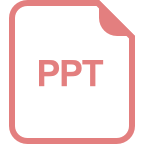
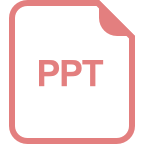