使用java代码实现将kv数据库和文档数据库作为alluxio数据源
时间: 2024-02-16 08:04:05 浏览: 111
在Java中使用Alluxio连接KV数据库和文档数据库作为数据源,需要实现Alluxio提供的自定义数据源接口。下面以Redis和MongoDB为例,介绍如何实现将它们作为Alluxio数据源。
1. Redis作为Alluxio数据源
首先需要引入Alluxio和Jedis的依赖:
```
<dependency>
<groupId>org.apache.alluxio</groupId>
<artifactId>alluxio-core-client</artifactId>
<version>${alluxio.version}</version>
</dependency>
<dependency>
<groupId>redis.clients</groupId>
<artifactId>jedis</artifactId>
<<version>2.9.0</version>
</dependency>
```
然后实现Alluxio提供的自定义数据源接口:
```
public class RedisAlluxioDataSource implements AlluxioURIPathTranslator, AlluxioStorageTypePathTranslator {
private final String redisHost;
private final int redisPort;
private final JedisPool jedisPool;
public RedisAlluxioDataSource(String redisHost, int redisPort) {
this.redisHost = redisHost;
this.redisPort = redisPort;
this.jedisPool = new JedisPool(redisHost, redisPort);
}
@Override
public AlluxioURI translate(AlluxioURI uri) {
String path = uri.getPath().substring(1); // 去掉路径前面的斜杠
try (Jedis jedis = jedisPool.getResource()) {
byte[] data = jedis.get(path.getBytes());
if (data != null) {
// 将Redis中的数据写入到Alluxio中
try (FileOutStream out = AlluxioFileSystem.Factory.get().createFile(uri)) {
out.write(data);
}
return uri;
}
} catch (Exception e) {
e.printStackTrace();
}
return null;
}
@Override
public StorageType translate(AlluxioURI uri) {
return StorageType.STORE; // 将所有数据都存储到Alluxio中
}
}
```
上面的代码实现了将Redis中的数据写入到Alluxio中,并返回Alluxio的URI。可以通过AlluxioFileSystem的createFile方法来创建Alluxio文件。
2. MongoDB作为Alluxio数据源
同样需要引入Alluxio和MongoDB的依赖:
```
<dependency>
<groupId>org.apache.alluxio</groupId>
<artifactId>alluxio-core-client</artifactId>
<version>${alluxio.version}</version>
</dependency>
<dependency>
<groupId>org.mongodb</groupId>
<artifactId>mongo-java-driver</artifactId>
<version>3.12.7</version>
</dependency>
```
然后实现Alluxio提供的自定义数据源接口:
```
public class MongoAlluxioDataSource implements AlluxioURIPathTranslator, AlluxioStorageTypePathTranslator {
private final String mongoHost;
private final int mongoPort;
private final String dbName;
private final String collectionName;
private final MongoClient mongoClient;
public MongoAlluxioDataSource(String mongoHost, int mongoPort, String dbName, String collectionName) {
this.mongoHost = mongoHost;
this.mongoPort = mongoPort;
this.dbName = dbName;
this.collectionName = collectionName;
this.mongoClient = new MongoClient(mongoHost, mongoPort);
}
@Override
public AlluxioURI translate(AlluxioURI uri) {
String path = uri.getPath().substring(1); // 去掉路径前面的斜杠
MongoCollection<Document> collection = mongoClient.getDatabase(dbName).getCollection(collectionName);
Document document = collection.find(eq("_id", path)).first();
if (document != null) {
// 将MongoDB中的数据写入到Alluxio中
try (FileOutStream out = AlluxioFileSystem.Factory.get().createFile(uri)) {
out.write(document.toJson().getBytes());
}
return uri;
}
return null;
}
@Override
public StorageType translate(AlluxioURI uri) {
return StorageType.STORE; // 将所有数据都存储到Alluxio中
}
}
```
上面的代码实现了将MongoDB中的数据写入到Alluxio中,并返回Alluxio的URI。同样可以通过AlluxioFileSystem的createFile方法来创建Alluxio文件。
最后需要将自定义的数据源注册到Alluxio中:
```
AlluxioURIPathTranslatorCache.addTranslator(new RedisAlluxioDataSource("127.0.0.1", 6379));
AlluxioStorageTypePathTranslatorCache.addTranslator(new RedisAlluxioDataSource("127.0.0.1", 6379));
AlluxioURIPathTranslatorCache.addTranslator(new MongoAlluxioDataSource("127.0.0.1", 27017, "test", "collection"));
AlluxioStorageTypePathTranslatorCache.addTranslator(new MongoAlluxioDataSource("127.0.0.1", 27017, "test", "collection"));
```
以上就是使用Java代码将KV数据库和文档数据库作为Alluxio数据源的方法。
阅读全文
相关推荐
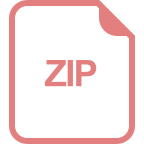
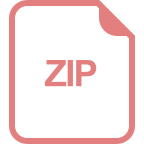
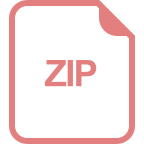

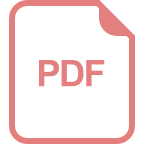
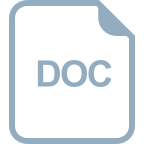
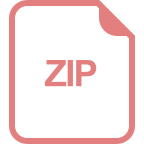
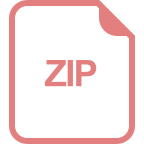
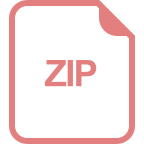
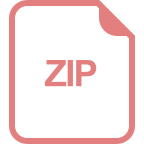
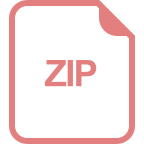
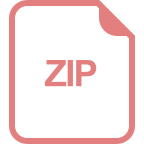
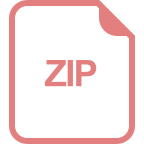
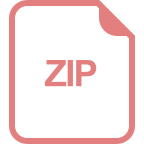
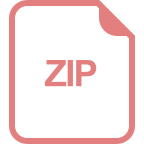
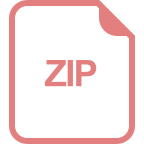