使用qt实现象棋棋子的功能
时间: 2023-12-15 21:07:12 浏览: 86
好的,实现象棋棋子的功能需要以下步骤:
1. 创建棋子类。可以创建一个 ChessPiece 类,用于存储棋子的颜色、类型、位置等信息。
2. 绘制棋子。在棋盘上绘制棋子,可以使用 Qt 中的 QPainter 进行绘制,根据棋子的类型和位置,绘制不同的图形。
3. 移动棋子。实现棋子的移动,可以在点击某个棋子时,记录下该棋子的位置,并生成一个移动棋子的提示框,根据鼠标移动的距离来移动棋子。
4. 判断棋子移动是否合法。根据象棋规则,判断棋子移动是否合法,可以根据棋子的类型和位置,结合棋局情况进行判断。
以下是一个简单的实现棋子的示例代码:
```cpp
// ChessPiece.h
#ifndef CHESSPIECE_H
#define CHESSPIECE_H
#include <QPoint>
#include <QString>
class ChessPiece
{
public:
enum Type { Ji, Ju, Ma, Xiang, Shi, Jiang, Pao };
enum Color { Red, Black };
ChessPiece(Type type, Color color, const QPoint& pos);
Type type() const;
Color color() const;
QPoint pos() const;
private:
Type m_type;
Color m_color;
QPoint m_pos;
};
#endif // CHESSPIECE_H
// ChessPiece.cpp
#include "ChessPiece.h"
ChessPiece::ChessPiece(Type type, Color color, const QPoint& pos)
: m_type(type), m_color(color), m_pos(pos)
{
}
ChessPiece::Type ChessPiece::type() const
{
return m_type;
}
ChessPiece::Color ChessPiece::color() const
{
return m_color;
}
QPoint ChessPiece::pos() const
{
return m_pos;
}
// ChessBoard.h
#ifndef CHESSBOARD_H
#define CHESSBOARD_H
#include <QWidget>
#include <QVector>
#include "ChessPiece.h"
class ChessBoard : public QWidget
{
Q_OBJECT
public:
ChessBoard(QWidget* parent = nullptr);
protected:
void paintEvent(QPaintEvent* event) override;
void mousePressEvent(QMouseEvent* event) override;
void mouseMoveEvent(QMouseEvent* event) override;
void mouseReleaseEvent(QMouseEvent* event) override;
private:
void drawChessBoard(QPainter& painter);
void drawChessPiece(QPainter& painter, const ChessPiece& piece);
int getPieceIndex(const QPoint& pos) const;
bool isMoveValid(const ChessPiece& piece, const QPoint& toPos) const;
private:
QVector<ChessPiece> m_redPieces;
QVector<ChessPiece> m_blackPieces;
int m_selectedIndex;
QPoint m_startPos;
QPoint m_endPos;
};
#endif // CHESSBOARD_H
// ChessBoard.cpp
#include "ChessBoard.h"
#include <QPainter>
#include <QMouseEvent>
ChessBoard::ChessBoard(QWidget* parent)
: QWidget(parent), m_selectedIndex(-1)
{
// 初始化棋子
m_redPieces.append(ChessPiece(ChessPiece::Ju, ChessPiece::Red, QPoint(0, 0)));
m_redPieces.append(ChessPiece(ChessPiece::Ma, ChessPiece::Red, QPoint(1, 0)));
m_redPieces.append(ChessPiece(ChessPiece::Xiang, ChessPiece::Red, QPoint(2, 0)));
m_redPieces.append(ChessPiece(ChessPiece::Shi, ChessPiece::Red, QPoint(3, 0)));
m_redPieces.append(ChessPiece(ChessPiece::Jiang, ChessPiece::Red, QPoint(4, 0)));
m_redPieces.append(ChessPiece(ChessPiece::Shi, ChessPiece::Red, QPoint(5, 0)));
m_redPieces.append(ChessPiece(ChessPiece::Xiang, ChessPiece::Red, QPoint(6, 0)));
m_redPieces.append(ChessPiece(ChessPiece::Ma, ChessPiece::Red, QPoint(7, 0)));
m_redPieces.append(ChessPiece(ChessPiece::Ju, ChessPiece::Red, QPoint(8, 0)));
m_redPieces.append(ChessPiece(ChessPiece::Pao, ChessPiece::Red, QPoint(1, 2)));
m_redPieces.append(ChessPiece(ChessPiece::Pao, ChessPiece::Red, QPoint(7, 2)));
m_blackPieces.append(ChessPiece(ChessPiece::Ju, ChessPiece::Black, QPoint(0, 9)));
m_blackPieces.append(ChessPiece(ChessPiece::Ma, ChessPiece::Black, QPoint(1, 9)));
m_blackPieces.append(ChessPiece(ChessPiece::Xiang, ChessPiece::Black, QPoint(2, 9)));
m_blackPieces.append(ChessPiece(ChessPiece::Shi, ChessPiece::Black, QPoint(3, 9)));
m_blackPieces.append(ChessPiece(ChessPiece::Jiang, ChessPiece::Black, QPoint(4, 9)));
m_blackPieces.append(ChessPiece(ChessPiece::Shi, ChessPiece::Black, QPoint(5, 9)));
m_blackPieces.append(ChessPiece(ChessPiece::Xiang, ChessPiece::Black, QPoint(6, 9)));
m_blackPieces.append(ChessPiece(ChessPiece::Ma, ChessPiece::Black, QPoint(7, 9)));
m_blackPieces.append(ChessPiece(ChessPiece::Ju, ChessPiece::Black, QPoint(8, 9)));
m_blackPieces.append(ChessPiece(ChessPiece::Pao, ChessPiece::Black, QPoint(1, 7)));
m_blackPieces.append(ChessPiece(ChessPiece::Pao, ChessPiece::Black, QPoint(7, 7)));
}
void ChessBoard::paintEvent(QPaintEvent* event)
{
QPainter painter(this);
drawChessBoard(painter);
for (const auto& piece : m_redPieces) {
drawChessPiece(painter, piece);
}
for (const auto& piece : m_blackPieces) {
drawChessPiece(painter, piece);
}
}
void ChessBoard::mousePressEvent(QMouseEvent* event)
{
if (event->button() == Qt::LeftButton) {
const auto index = getPieceIndex(event->pos());
if (index >= 0) {
m_selectedIndex = index;
m_startPos = event->pos();
}
}
}
void ChessBoard::mouseMoveEvent(QMouseEvent* event)
{
if (m_selectedIndex >= 0) {
m_endPos = event->pos();
update();
}
}
void ChessBoard::mouseReleaseEvent(QMouseEvent* event)
{
if (event->button() == Qt::LeftButton && m_selectedIndex >= 0) {
const auto toPos = event->pos();
if (isMoveValid(m_redPieces[m_selectedIndex], toPos)) {
m_redPieces[m_selectedIndex].pos() = toPos;
}
m_selectedIndex = -1;
update();
}
}
void ChessBoard::drawChessBoard(QPainter& painter)
{
// 绘制棋盘
}
void ChessBoard::drawChessPiece(QPainter& painter, const ChessPiece& piece)
{
// 根据棋子信息绘制棋子
}
int ChessBoard::getPieceIndex(const QPoint& pos) const
{
// 根据鼠标点击位置获取对应的棋子编号
}
bool ChessBoard::isMoveValid(const ChessPiece& piece, const QPoint& toPos) const
{
// 判断棋子移动是否合法
}
```
以上代码实现了象棋棋子的基本功能,包括棋子的绘制、移动和判断移动是否合法。具体实现细节可以根据实际需求进行调整。
阅读全文
相关推荐




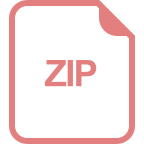
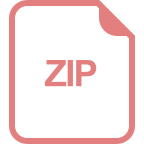
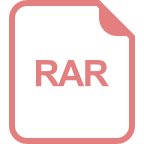
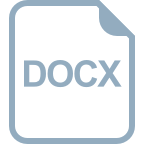
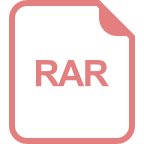
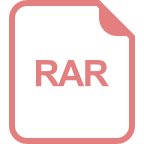
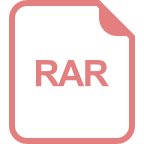
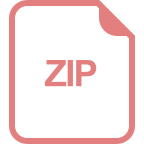
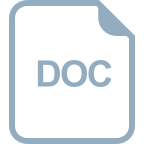
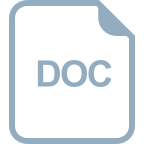
