将faster rcnn中的smooth l1损失函数修改为iou loss损失函数,并给出代码
时间: 2024-03-11 10:48:10 浏览: 23
将 Faster R-CNN 中的 smooth L1 loss 修改为 IoU loss 可以尝试如下代码实现:
```python
import torch
def iou_loss(pred_bbox, gt_bbox, eps=1e-6):
"""
Compute IoU loss between predicted bboxes and ground truth bboxes.
Args:
pred_bbox: predicted bboxes, shape [N, 4]
gt_bbox: ground truth bboxes, shape [N, 4]
eps: epsilon to avoid divide by zero
Returns:
iou_loss: IoU loss between predicted bboxes and ground truth bboxes, shape [N]
"""
# compute IoU
x1 = torch.max(pred_bbox[:, 0], gt_bbox[:, 0])
y1 = torch.max(pred_bbox[:, 1], gt_bbox[:, 1])
x2 = torch.min(pred_bbox[:, 2], gt_bbox[:, 2])
y2 = torch.min(pred_bbox[:, 3], gt_bbox[:, 3])
w = torch.clamp(x2 - x1, min=0)
h = torch.clamp(y2 - y1, min=0)
inter = w * h
a1 = (pred_bbox[:, 2] - pred_bbox[:, 0]) * (pred_bbox[:, 3] - pred_bbox[:, 1])
a2 = (gt_bbox[:, 2] - gt_bbox[:, 0]) * (gt_bbox[:, 3] - gt_bbox[:, 1])
union = a1 + a2 - inter
iou = inter / (union + eps)
# compute IoU loss
threshold = 0.5
iou_loss = torch.pow(iou - threshold, 2)
return iou_loss
# example usage
pred_bbox = torch.tensor([[2.0, 3.0, 5.0, 6.0], [1.0, 2.0, 4.0, 5.0]])
gt_bbox = torch.tensor([[1.0, 2.0, 4.0, 5.0], [2.0, 3.0, 5.0, 6.0]])
loss = iou_loss(pred_bbox, gt_bbox)
print(loss)
```
然后将 Faster R-CNN 中的 smooth L1 loss 替换为 iou loss,如下所示:
```python
import torch
import torch.nn as nn
def iou_loss(pred_bbox, gt_bbox, eps=1e-6):
"""
Compute IoU loss between predicted bboxes and ground truth bboxes.
Args:
pred_bbox: predicted bboxes, shape [N, 4]
gt_bbox: ground truth bboxes, shape [N, 4]
eps: epsilon to avoid divide by zero
Returns:
iou_loss: IoU loss between predicted bboxes and ground truth bboxes, shape [N]
"""
# compute IoU
x1 = torch.max(pred_bbox[:, 0], gt_bbox[:, 0])
y1 = torch.max(pred_bbox[:, 1], gt_bbox[:, 1])
x2 = torch.min(pred_bbox[:, 2], gt_bbox[:, 2])
y2 = torch.min(pred_bbox[:, 3], gt_bbox[:, 3])
w = torch.clamp(x2 - x1, min=0)
h = torch.clamp(y2 - y1, min=0)
inter = w * h
a1 = (pred_bbox[:, 2] - pred_bbox[:, 0]) * (pred_bbox[:, 3] - pred_bbox[:, 1])
a2 = (gt_bbox[:, 2] - gt_bbox[:, 0]) * (gt_bbox[:, 3] - gt_bbox[:, 1])
union = a1 + a2 - inter
iou = inter / (union + eps)
# compute IoU loss
threshold = 0.5
iou_loss = torch.pow(iou - threshold, 2)
return iou_loss.mean()
class FasterRCNN(nn.Module):
def __init__(self, num_classes):
super().__init__()
self.num_classes = num_classes
self.backbone = ...
self.rpn = ...
self.roi_head = ...
self.bbox_head = nn.Linear(4096, 4 * self.num_classes)
self.cls_head = nn.Linear(4096, self.num_classes)
def forward(self, x, gt_bbox=None):
# backbone
x = self.backbone(x)
# RPN
rpn_cls, rpn_bbox = self.rpn(x)
# RoI pooling
rois = self.roi_head(x, rpn_bbox)
# bbox regression
bbox_pred = self.bbox_head(rois)
bbox_pred = bbox_pred.reshape(-1, 4)
# classification
cls_score = self.cls_head(rois)
cls_score = cls_score.reshape(-1, self.num_classes)
cls_prob = nn.functional.softmax(cls_score, dim=1)
# test or train
if self.training:
# compute loss
rpn_loss, roi_loss = ...
bbox_loss = iou_loss(bbox_pred, gt_bbox)
cls_loss = ...
total_loss = rpn_loss + roi_loss + bbox_loss + cls_loss
return total_loss
else:
# inference
result = ...
return result
```
需要注意的是,IoU loss 可能会导致梯度爆炸或梯度消失的问题,因此需要进行一些处理,例如使用渐进式策略或者加入正则化项等。
相关推荐
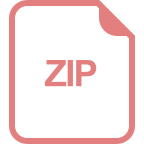
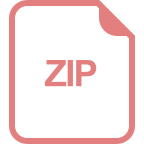
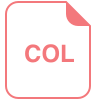













