python数据库连接
时间: 2023-10-12 22:15:09 浏览: 97
在 Python 中连接数据库的方式有很多种,以下是常用的几种:
1. 使用 MySQL 数据库
```
import mysql.connector
# 连接数据库
mydb = mysql.connector.connect(
host="localhost",
user="yourusername",
password="yourpassword",
database="yourdatabase"
)
# 查询
mycursor = mydb.cursor()
mycursor.execute("SELECT * FROM yourtable")
myresult = mycursor.fetchall()
for x in myresult:
print(x)
```
2. 使用 SQLite 数据库
```
import sqlite3
# 连接数据库
conn = sqlite3.connect('example.db')
# 查询
c = conn.cursor()
c.execute("SELECT * FROM yourtable")
rows = c.fetchall()
for row in rows:
print(row)
```
3. 使用 PostgreSQL 数据库
```
import psycopg2
# 连接数据库
conn = psycopg2.connect(
host="localhost",
database="yourdatabase",
user="yourusername",
password="yourpassword"
)
# 查询
cur = conn.cursor()
cur.execute("SELECT * FROM yourtable")
rows = cur.fetchall()
for row in rows:
print(row)
```
以上是三种常用的 Python 数据库连接方式,具体使用哪一种取决于你的项目需求和数据库类型。
阅读全文
相关推荐
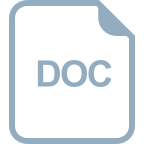
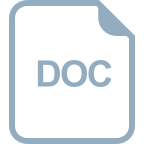
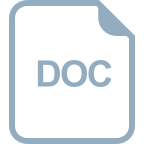
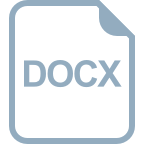
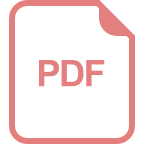
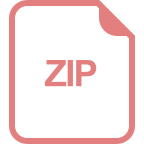
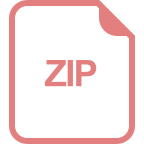
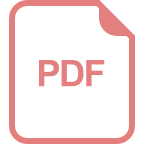
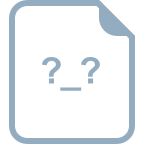
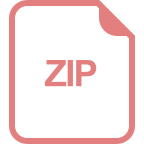
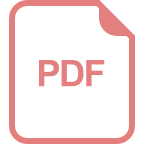
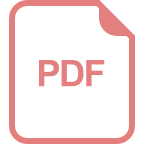
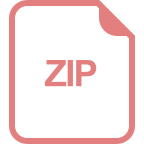
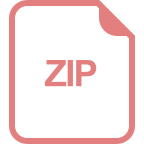
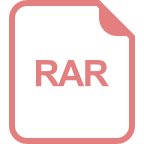
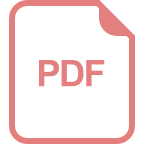