如何在codeblock上实现以下代码SeqList *inti_SeqList(){ SeqList *L;L=malloc(sizeof(SeqList));L->last=-1;return L;}
时间: 2023-12-06 16:44:20 浏览: 90
可以按照以下步骤在Code::Blocks上实现该代码:
1. 打开Code::Blocks,创建一个新的C文件。
2. 在文件中输入以下代码:
```
#include <stdlib.h>
#include <stdio.h>
typedef struct SeqList{
int last;
}SeqList;
SeqList *inti_SeqList(){
SeqList *L;
L=malloc(sizeof(SeqList));
L->last=-1;
return L;
}
int main(){
SeqList *list = inti_SeqList();
printf("The last element is: %d\n", list->last);
return 0;
}
```
3. 点击菜单栏上的“Build and Run”按钮进行编译和运行。
4. 程序将输出“The last element is: -1”,表示成功创建了一个空的顺序表。
5. 注意:代码中的“SeqList”是自定义的结构体类型,其定义在代码的开头。函数“inti_SeqList()”用于初始化该结构体,并返回指向该结构体的指针。
相关问题
如何在CodeBlock中实现自定义排序规则?
在CodeBlock中实现自定义排序规则通常涉及两个步骤:定义排序函数和应用该函数到你要排序的数据上。这里以几种常见编程语言为例:
1. **Python**:
```python
def custom_sort(data, key_func):
data.sort(key=key_func)
return data
def my_custom_rule(item): # 定义按字符串长度排序的函数
return len(item)
list_to_sort = ["apple", "banana", "cherry"]
sorted_list = custom_sort(list_to_sort, my_custom_rule)
```
2. **JavaScript**:
```javascript
function customSort(array, compareFunc) {
array.sort(compareFunc);
return array;
}
function customRule(item) { // 定义按数值大小排序的函数
return item;
}
const arrayToSort = [4, 2, 7, 1, 9];
const sortedArray = customSort(arrayToSort, customRule);
```
3. **Java (Comparator)**:
```java
List<String> list = new ArrayList<>();
// ...
Collections.sort(list, new Comparator<String>() {
public int compare(String a, String b) {
return a.length() - b.length(); // 自定义排序规则
}
});
```
在上述代码中,`key_func`或`compareFunc`就是你的自定义排序规则,它可以基于任何你想要的标准(如字符串长度、数字大小等)来决定元素之间的顺序。根据你的需求,只需替换相应的函数即可。
用codeblock写一个关于sizeof运算符的代码
当然,下面是一个简单的代码示例,展示了如何在 C 语言中使用 `sizeof` 运算符来获取不同类型的数据以及数组的大小:
```c
#include <stdio.h>
// 定义基本数据类型
int iValue;
float fValue;
// 定义结构体
struct Student {
char name[50];
int age;
};
int main() {
// 测量基本数据类型大小
printf("Size of an integer: %zu bytes\n", sizeof(iValue));
printf("Size of a float: %zu bytes\n", sizeof(fValue));
// 测量数组大小
int arr[10];
printf("Size of the integer array: %zu bytes\n", sizeof(arr));
// 测量结构体大小
struct Student s;
printf("Size of a Student struct: %zu bytes\n", sizeof(s));
return 0;
}
```
当你运行这个程序,它会输出每个变量和结构体所占用的字节数。注意,这里的 `sizeof` 返回的是字节数,而不是元素的数量。
阅读全文
相关推荐






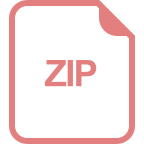





