串行序列信号接收器VHDL
时间: 2023-08-03 11:38:44 浏览: 42
串行序列信号接收器的VHDL实现可以分为以下几个步骤:
1. 定义输入和输出端口
在VHDL代码中,需要定义输入和输出端口。对于串行序列信号接收器来说,输入端口通常包括一个时钟信号、一个数据信号和一个复位信号;输出端口包括一个输出数据信号和一个有效信号。代码示例如下:
```
entity serial_receiver is
port (
clk : in std_logic; -- 时钟信号
data_in : in std_logic; -- 数据信号
reset : in std_logic; -- 复位信号
data_out : out std_logic; -- 输出数据信号
valid : out std_logic -- 有效信号
);
end serial_receiver;
```
2. 定义内部信号
在VHDL代码中,需要定义一些内部信号来存储和处理输入数据。对于串行序列信号接收器来说,需要定义一个计数器来计算接收到的数据位数,以及一个移位寄存器来存储接收到的数据。代码示例如下:
```
architecture Behavioral of serial_receiver is
signal count : integer := 0; -- 计数器
signal shift_reg : std_logic_vector(7 downto 0); -- 移位寄存器
begin
...
end Behavioral;
```
3. 编写状态机
串行序列信号接收器的VHDL实现通常使用状态机来处理输入数据。状态机包含多个状态,根据输入信号和当前状态转移到下一个状态。状态机的实现方式有多种,这里以 Mealy 状态机为例。代码示例如下:
```
...
-- 状态机
process (clk, reset)
begin
if reset = '1' then -- 复位
state <= IDLE;
count <= 0;
data_out <= '0';
valid <= '0';
elsif rising_edge(clk) then -- 上升沿触发
case state is
when IDLE => -- 空闲状态
if data_in = '0' then
state <= START;
else
state <= IDLE;
end if;
when START => -- 接收起始位
if count < 8 then
shift_reg <= shift_reg(6 downto 0) & data_in;
count <= count + 1;
state <= DATA;
else
state <= IDLE;
end if;
when DATA => -- 接收数据位
if count < 8 then
shift_reg <= shift_reg(6 downto 0) & data_in;
count <= count + 1;
state <= DATA;
else
state <= STOP;
end if;
when STOP => -- 接收停止位
if data_in = '1' then
data_out <= shift_reg;
valid <= '1';
state <= IDLE;
else
state <= IDLE;
end if;
end case;
end if;
end process;
...
```
4. 输出结果
最后,需要在状态机中根据接收到的数据计算输出结果,并将输出数据和有效信号输出到端口。代码示例如下:
```
...
-- 输出结果
data_out <= shift_reg;
valid <= '1' when state = STOP else '0';
...
```
完整的串行序列信号接收器的VHDL代码示例如下:
```
entity serial_receiver is
port (
clk : in std_logic;
data_in : in std_logic;
reset : in std_logic;
data_out : out std_logic;
valid : out std_logic
);
end serial_receiver;
architecture Behavioral of serial_receiver is
signal count : integer := 0;
signal shift_reg : std_logic_vector(7 downto 0);
type state_type is (IDLE, START, DATA, STOP);
signal state : state_type := IDLE;
begin
-- 状态机
process (clk, reset)
begin
if reset = '1' then -- 复位
state <= IDLE;
count <= 0;
data_out <= '0';
valid <= '0';
elsif rising_edge(clk) then -- 上升沿触发
case state is
when IDLE => -- 空闲状态
if data_in = '0' then
state <= START;
else
state <= IDLE;
end if;
when START => -- 接收起始位
if count < 8 then
shift_reg <= shift_reg(6 downto 0) & data_in;
count <= count + 1;
state <= DATA;
else
state <= IDLE;
end if;
when DATA => -- 接收数据位
if count < 8 then
shift_reg <= shift_reg(6 downto 0) & data_in;
count <= count + 1;
state <= DATA;
else
state <= STOP;
end if;
when STOP => -- 接收停止位
if data_in = '1' then
data_out <= shift_reg;
valid <= '1';
state <= IDLE;
else
state <= IDLE;
end if;
end case;
end if;
end process;
-- 输出结果
data_out <= shift_reg;
valid <= '1' when state = STOP else '0';
end Behavioral;
```
相关推荐
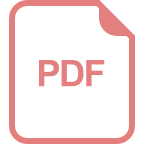
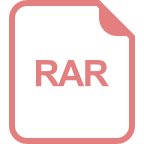














