用convas和vue及element-ui实现一个任意图像,用具体代码实现
时间: 2024-01-21 16:19:44 浏览: 146
要使用 Canvas 和 Vue 实现一个任意图像,需要进行以下步骤:
1. 创建一个 Canvas 元素
在 Vue 组件中,可以使用 `ref` 属性来引用一个元素。在模板中添加一个 `canvas` 标签,并设置 `ref` 属性:
```html
<template>
<div>
<canvas ref="canvas"></canvas>
</div>
</template>
```
在组件的 `mounted` 生命周期钩子中获取 Canvas 元素的上下文对象,并设置其宽高:
```javascript
mounted() {
const canvas = this.$refs.canvas;
const ctx = canvas.getContext('2d');
canvas.width = 500;
canvas.height = 500;
// 在这里可以使用 ctx 对象绘制图形
}
```
2. 绘制图像
可以使用 Canvas 上下文对象的方法来绘制图像。以下是一个简单的例子,绘制一个红色矩形和一个蓝色圆形:
```javascript
mounted() {
const canvas = this.$refs.canvas;
const ctx = canvas.getContext('2d');
canvas.width = 500;
canvas.height = 500;
// 绘制矩形
ctx.fillStyle = 'red';
ctx.fillRect(50, 50, 100, 100);
// 绘制圆形
ctx.fillStyle = 'blue';
ctx.beginPath();
ctx.arc(250, 250, 50, 0, Math.PI * 2);
ctx.fill();
}
```
3. 使用 Element UI 添加样式
可以使用 Element UI 提供的组件来添加样式,例如按钮、卡片等。以下是一个简单的例子,使用 Element UI 的卡片组件来包含 Canvas 元素:
```html
<template>
<div>
<el-card>
<canvas ref="canvas"></canvas>
</el-card>
</div>
</template>
```
可以在组件中导入 `el-card` 组件:
```javascript
import { Card } from 'element-ui';
export default {
components: {
'el-card': Card
},
// ...
}
```
完整的代码示例如下:
```html
<template>
<div>
<el-card>
<canvas ref="canvas"></canvas>
</el-card>
</div>
</template>
<script>
import { Card } from 'element-ui';
export default {
components: {
'el-card': Card
},
mounted() {
const canvas = this.$refs.canvas;
const ctx = canvas.getContext('2d');
canvas.width = 500;
canvas.height = 500;
// 绘制矩形
ctx.fillStyle = 'red';
ctx.fillRect(50, 50, 100, 100);
// 绘制圆形
ctx.fillStyle = 'blue';
ctx.beginPath();
ctx.arc(250, 250, 50, 0, Math.PI * 2);
ctx.fill();
}
}
</script>
```
阅读全文
相关推荐











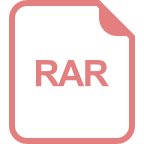





