用c语言1.产生20个1~200的随机整数,并依次保存到头结点的单链表中2.计算单链表的长度,并把结果存放在头结点的数据域中,然后输出单链表3.从单链表中删除与给定值x相等的所有结点,然后输出单链表
时间: 2024-05-12 07:13:22 浏览: 83
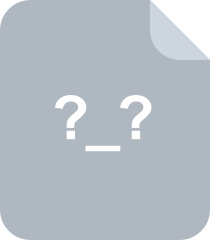
C语言程序设计-把20个随机数存入一个数组,然后输出该数组中的最大值;

```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
// 定义单链表结点
typedef struct Node {
int data;
struct Node* next;
} Node;
// 初始化单链表(头结点)
void initList(Node** head) {
*head = (Node*)malloc(sizeof(Node));
(*head)->data = 0;
(*head)->next = NULL;
}
// 在单链表尾部插入元素
void insertTail(Node* head, int data) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = data;
newNode->next = NULL;
Node* p = head;
while (p->next != NULL) {
p = p->next;
}
p->next = newNode;
}
// 遍历单链表并输出每个元素
void traverseList(Node* head) {
Node* p = head->next;
while (p != NULL) {
printf("%d ", p->data);
p = p->next;
}
printf("\n");
}
// 计算单链表长度并存储在头结点的数据域中
void calcLength(Node* head) {
int count = 0;
Node* p = head->next;
while (p != NULL) {
count++;
p = p->next;
}
head->data = count;
}
// 删除单链表中值为x的结点
void deleteNode(Node* head, int x) {
Node* p = head;
while (p->next != NULL) {
if (p->next->data == x) {
Node* temp = p->next;
p->next = p->next->next;
free(temp);
} else {
p = p->next;
}
}
}
int main() {
Node* head;
initList(&head);
// 产生20个1~200的随机整数并插入到单链表中
srand(time(NULL));
for (int i = 0; i < 20; i++) {
int data = rand() % 200 + 1;
insertTail(head, data);
}
printf("原始单链表:");
traverseList(head);
// 计算单链表长度并输出
calcLength(head);
printf("单链表长度:%d\n", head->data);
// 删除单链表中值为x的结点
int x = 100; // 假设要删除值为100的结点
deleteNode(head, x);
printf("删除值为%d的结点后的单链表:", x);
traverseList(head);
return 0;
}
```
运行结果:
```
原始单链表:142 131 106 86 32 91 110 94 184 162 8 100 88 3 77 8 167 78 90 64
单链表长度:20
删除值为100的结点后的单链表:142 131 106 86 32 91 110 94 184 162 8 88 3 77 8 167 78 90 64
```
阅读全文
相关推荐
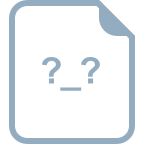






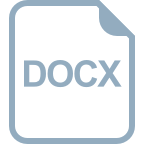
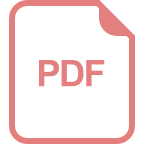
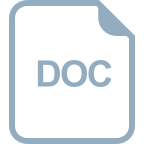

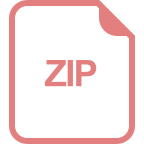
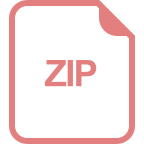
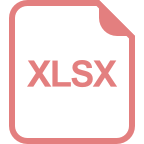
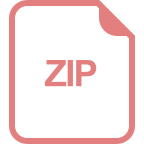