用C#写个猫和狗的宠物管理控制台程序
时间: 2023-08-10 17:17:57 浏览: 69
好的,以下是一个简单的猫和狗的宠物管理控制台程序的示例代码,使用C#编写。程序可以添加、删除、列出宠物信息等操作。
```csharp
using System;
using System.Collections.Generic;
namespace PetManagement
{
class Program
{
static List<Pet> pets = new List<Pet>();
static void Main(string[] args)
{
while (true)
{
Console.WriteLine("请选择操作:");
Console.WriteLine("1. 添加宠物");
Console.WriteLine("2. 删除宠物");
Console.WriteLine("3. 列出宠物信息");
Console.WriteLine("4. 退出");
string choice = Console.ReadLine();
switch (choice)
{
case "1":
AddPet();
break;
case "2":
RemovePet();
break;
case "3":
ListPets();
break;
case "4":
Environment.Exit(0);
break;
default:
Console.WriteLine("无效的选择,请重新输入。");
break;
}
}
}
static void AddPet()
{
Console.WriteLine("请输入宠物类型(cat/dog):");
string type = Console.ReadLine();
Console.WriteLine("请输入宠物名称:");
string name = Console.ReadLine();
if (type == "cat")
{
pets.Add(new Cat(name));
Console.WriteLine("添加成功。");
}
else if (type == "dog")
{
pets.Add(new Dog(name));
Console.WriteLine("添加成功。");
}
else
{
Console.WriteLine("无效的宠物类型。");
}
}
static void RemovePet()
{
Console.WriteLine("请输入要删除的宠物名称:");
string name = Console.ReadLine();
bool removed = false;
foreach (Pet pet in pets)
{
if (pet.Name == name)
{
pets.Remove(pet);
Console.WriteLine("删除成功。");
removed = true;
break;
}
}
if (!removed)
{
Console.WriteLine("找不到要删除的宠物。");
}
}
static void ListPets()
{
Console.WriteLine("宠物列表:");
foreach (Pet pet in pets)
{
Console.WriteLine("{0} - {1}", pet.Type, pet.Name);
}
}
}
abstract class Pet
{
public string Type { get; protected set; }
public string Name { get; protected set; }
public Pet(string type, string name)
{
Type = type;
Name = name;
}
}
class Cat : Pet
{
public Cat(string name) : base("cat", name)
{
}
}
class Dog : Pet
{
public Dog(string name) : base("dog", name)
{
}
}
}
```
程序中定义了一个`Pet`抽象类,表示宠物的基本信息。`Cat`和`Dog`类继承自`Pet`类,分别表示猫和狗。在`Program`类中,定义了一个静态的`List<Pet>`对象`pets`,表示存储所有宠物的列表。
程序的主要逻辑在`Main`方法中,使用无限循环来等待用户输入操作,根据用户的不同选择执行不同的方法。`AddPet`方法用于添加新宠物,根据用户输入的类型和名称创建新的`Cat`或`Dog`对象,并添加到`pets`列表中。`RemovePet`方法用于删除已有宠物,根据用户输入的名称查找对应的宠物,并从`pets`列表中删除它。`ListPets`方法用于列出所有宠物的信息,遍历`pets`列表并输出每个宠物的类型和名称。
以上是一个简单的猫和狗的宠物管理控制台程序的示例代码,仅供参考。
相关推荐
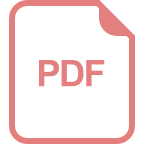














