用52单片机写一个DS18B20的程序
时间: 2023-05-21 13:04:58 浏览: 63
好的,以下是一个基于52单片机的DS18B20温度传感器读取程序:
```c
#include<reg52.h>
#include<intrins.h>
#define uchar unsigned char
#define uint unsigned int
sbit DQ=P2^0;
// 延时函数,x为延时的微秒数
void DelayXus(uint x) {
while(x--);
}
// 向DS18B20写一个字节
void WriteByte(uchar dat) {
uchar i;
for(i=0;i<8;i++) {
DQ=0;
_nop_();
DQ=dat&0x01;
_nop_();
DQ=1;
dat>>=1;
}
}
// 从DS18B20读一个字节
uchar ReadByte() {
uchar i,dat;
for(i=0;i<8;i++) {
DQ=0;
_nop_();
dat>>=1;
if(DQ) dat|=0x80;
_nop_();
DQ=1;
}
return dat;
}
// 初始化DS18B20
uchar Init_DS18B20() {
uchar i;
DQ=1;
DelayXus(2);
DQ=0;
DelayXus(80);
DQ=1;
DelayXus(10);
i=DQ;
DelayXus(50);
return i;
}
// 对DS18B20发出读指令
void ReadTemperature() {
Init_DS18B20();
WriteByte(0xcc);
WriteByte(0x44);
}
// 对DS18B20发出读温度命令
void ReadTempCom() {
Init_DS18B20();
WriteByte(0xcc);
WriteByte(0xbe);
}
// 读取温度数据
int ReadTempData() {
uchar low,high;
int temp;
ReadTempCom();
low=ReadByte();
high=ReadByte();
temp=(high<<8)+low;
return temp;
}
void main() {
int temp;
while(1) {
ReadTemperature();
DelayXus(100);
temp=ReadTempData();
// 将读到的温度数据temp转化为实际温度
temp=temp*0.0625;
// 显示温度(假设用了I2C的液晶屏)
// ShowTemperature(temp);
}
}
```
注意,实际应用中还需要在读取温度数据前对DS18B20进行复位和初始化等操作。
相关推荐
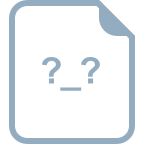














