如何用C#开发一个 对欧姆龙NJ501 OPCUA读写的C# 程序
时间: 2023-12-01 22:00:47 浏览: 133
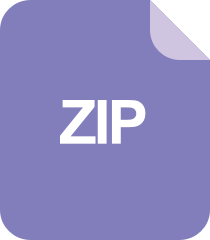
C#基于OPC服务器读写欧姆龙PLC数据实例源码

要用C#开发一个对欧姆龙NJ501 OPCUA读写的C#程序,可以遵循以下步骤:
1. 安装NuGet包管理器。在Visual Studio中,右键单击项目,选择“管理NuGet程序包”,搜索“Opc.Ua”并安装。
2. 创建OPCUA客户端。使用Opc.Ua.Client命名空间,创建一个OPCUA客户端实例。通过OPCUA客户端实例,可以连接到远程OPCUA服务器。
3. 连接到远程OPCUA服务器。使用OPCUA客户端实例的Connect方法连接到远程OPCUA服务器。需要指定OPCUA服务器的IP地址和端口号。
4. 浏览OPCUA服务器。使用OPCUA客户端实例的Browse方法,可以浏览OPCUA服务器的节点和对象。
5. 读取OPCUA变量。使用OPCUA客户端实例的Read方法,可以读取OPCUA服务器的变量的值。
6. 写入OPCUA变量。使用OPCUA客户端实例的Write方法,可以写入OPCUA服务器的变量的值。
下面是一个简单的示例,演示如何使用C#代码读取和写入OPCUA变量:
```csharp
using System;
using Opc.Ua;
using Opc.Ua.Client;
// 创建OPCUA客户端
var endpointUrl = "opc.tcp://localhost:4840"; // 远程OPCUA服务器的地址
var client = new Opc.Ua.Client.Session(new ConfiguredEndpoint(null, new EndpointDescription(endpointUrl), EndpointConfiguration.Create()));
// 连接到远程OPCUA服务器
client.Connect();
// 浏览OPCUA服务器
var root = client.Browse(ExpandedNodeId.ToNodeId(ObjectIds.ObjectsFolder, client.NamespaceUris));
foreach (var node in root)
{
Console.WriteLine(node.DisplayName);
}
// 读取OPCUA变量
var nodeToRead = new ReadValueId { NodeId = new NodeId("ns=2;s=MyVariable"), AttributeId = Attributes.Value };
var result = client.Read(null, 0, TimestampsToReturn.Both, nodeToRead);
Console.WriteLine($"读取值:{result.Value}");
// 写入OPCUA变量
var nodeToWrite = new WriteValue { NodeId = new NodeId("ns=2;s=MyVariable"), AttributeId = Attributes.Value, Value = new DataValue(42) };
client.Write(null, nodeToWrite);
```
需要注意的是,在读取和写入OPCUA变量时,需要确保节点的ID和命名空间与OPCUA服务器中的节点匹配。
阅读全文
相关推荐
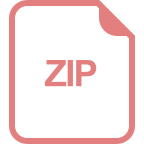
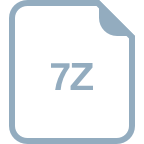
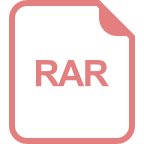
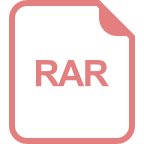
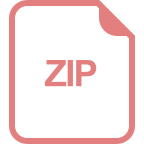
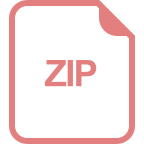
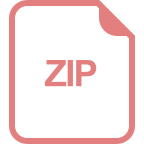
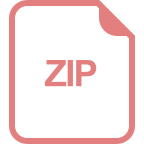
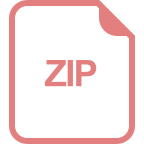
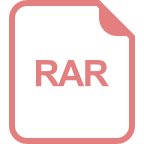
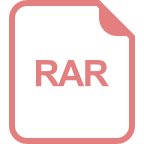
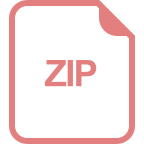
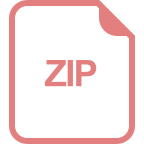
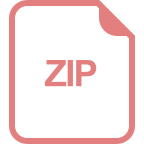