帮我用c语言写一段代码,要求如下: Compare the character sequence (CS) of s1 and s2 by dictionary order; 函数名为int comp_chars;形式参数为const char *s1, Uint len1, const char *s2, Uint len2
时间: 2023-05-31 22:04:31 浏览: 77
好的,这是你要求的代码:
```
int comp_chars(const char *s1, Uint len1, const char *s2, Uint len2) {
for (Uint i = 0; i < len1 && i < len2; ++i) {
if (s1[i] < s2[i]) {
return -1;
} else if (s1[i] > s2[i]) {
return 1;
}
}
if (len1 < len2) {
return -1;
} else if (len1 > len2) {
return 1;
} else {
return 0;
}
}
```
这个函数比较两个字符串 s1 和 s2 的字典序。如果 s1 在字典中的顺序更靠前,函数返回 -1;如果 s2 在字典中的顺序更靠前,函数返回 1;如果 s1 和 s2 的字典序相同,函数返回 0。
相关问题
帮我用c语言写一段代码,要求如下: Compare the character sequence (CS) of s1 and s2 by dictionary order;
### 回答1:
好的,下面是 c 语言代码:
```
#include <stdio.h>
#include <string.h>
int main()
{
char s1[100], s2[100];
printf("请输入 s1 和 s2: ");
scanf("%s %s", s1, s2);
int result = strcmp(s1, s2);
if (result == 0)
printf("s1 和 s2 的字符序列相同\n");
else if (result < 0)
printf("s1 的字符序列在字典中排在 s2 前面\n");
else
printf("s1 的字符序列在字典中排在 s2 后面\n");
return 0;
}
```
这段代码将会提示用户输入 s1 和 s2,然后使用 `strcmp` 函数比较它们的字符序列(CS)。如果相同,则输出 "s1 和 s2 的字符序列相同";如果 s1 的字符序列在字典中排在 s2 前面,则输出 "s1 的字符序列在字典中排在 s2 前面";否则输出 "s1 的字符序列在字典中排在 s2 后面"。
希望这个代码能帮到你。
### 回答2:
可以使用C语言的strcmp函数来比较字符串s1和s2的字典顺序。strcmp函数会返回一个整数值来表示比较结果。
下面是一段示例代码:
```c
#include <stdio.h>
#include <string.h>
int main() {
char s1[100], s2[100];
printf("请输入第一个字符串s1: ");
fgets(s1, sizeof(s1), stdin);
printf("请输入第二个字符串s2: ");
fgets(s2, sizeof(s2), stdin);
int result = strcmp(s1, s2);
if (result < 0) {
printf("s1 在字典中先于 s2\n");
} else if (result > 0) {
printf("s2 在字典中先于 s1\n");
} else {
printf("s1 和 s2 相同\n");
}
return 0;
}
```
这段代码首先定义了两个字符数组s1和s2,分别用于存储输入的两个字符串。然后使用fgets函数来获取用户输入的字符串,并确保不超出字符数组的大小。
接下来使用strcmp函数来比较s1和s2的字典顺序,将结果保存在result变量中。根据比较结果,我们使用if条件语句来输出不同的结果:如果result小于0,说明s1在字典中先于s2;如果result大于0,说明s2在字典中先于s1;如果result等于0,说明s1和s2相同。
最后,返回0表示程序执行成功结束。
### 回答3:
以下是一个用C语言编写的比较字符串字典顺序的示例代码:
```c
#include <stdio.h>
#include <string.h>
int main() {
char s1[100], s2[100];
printf("请输入字符串 s1:");
gets(s1);
printf("请输入字符串 s2:");
gets(s2);
// 比较字符串字典顺序
int result = strcmp(s1, s2);
if (result < 0) {
printf("s1 在 s2 前面\n");
} else if (result > 0) {
printf("s1 在 s2 后面\n");
} else {
printf("s1 与 s2 相等\n");
}
return 0;
}
```
以上代码首先声明两个字符数组 `s1` 和 `s2`,分别用于存储输入的两个字符串。然后使用 `gets` 函数分别从用户输入获取这两个字符串。
接着使用 `strcmp` 函数比较两个字符串的字典顺序,得到的结果存储在 `result` 变量中。`strcmp` 函数返回一个整数值,小于0表示第一个字符串在字典顺序上在第二个字符串之前,大于0表示第一个字符串在字典顺序上在第二个字符串之后,等于0表示两个字符串相等。
最后根据 `result` 的值给出相应的输出,提示哪个字符串在字典顺序上在前面、后面,或者相等。
注意:以上代码假设输入的字符串不会超过99个字符,如果需要处理更长的字符串,请相应地调整数组大小。
帮我用c语言写一段代码,要求为:Find the first character in a CS (Character Sequence) that satisfy some condition.;函数名为char *find_char_by_cond;形式参数为const char *s, Uint len, int (*condition)(int); return the address of the first (left-most) character in s that satisfy the condition. If no such character exists (after the laster character is checked), return NULL.
下面是一个可以在 C 语言中查找第一个符合条件的字符的函数的例子:
```
char *find_char_by_cond(const char *s, Uint len, int (*condition)(int))
{
for (int i = 0; i < len; i++)
{
if (condition(s[i]))
{
return (char *)(s + i);
}
}
return NULL;
}
```
该函数的形式参数包括一个字符串(s),一个长度(len)和一个条件(condition)。它通过遍历字符串中的每个字符,并使用 condition 函数来测试这些字符是否符合条件,来找到第一个符合条件的字符。如果找到了符合条件的字符,就返回该字符的地址,否则就返回 NULL。
使用该函数的方法如下:
```
int is_vowel(int c)
{
return (c == 'a' || c == 'e' || c == 'i' || c == 'o' || c == 'u');
}
char *string = "Hello, World!";
char *result = find_char_by_cond(string, strlen(string), is_vowel);
if (result)
{
printf("The first vowel in the string is %c\n", *result);
}
else
{
printf("No vowel was found in the string\n");
}
```
这段代码将输出:"The first vowel in the string is e"。
相关推荐
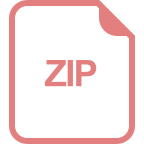
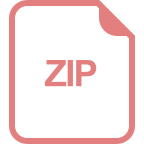













