public List<Doctor> query(String where, Object[] o){ List<Doctor> doctors=new ArrayList<>(); String sql="select * from doctor "+where; ResultSet rs=DBUtil.executeQuery(sql,o); try { while (rs.next()){ doctors.add(new Doctor(rs.getString(1), rs.getString(2), rs.getString(3), rs.getString(4), rs.getString(5), rs.getString(6), rs.getString(7), rs.getString(8), rs.getString(9), rs.getString(10), rs.getString(11))); } } catch (SQLException e) { e.printStackTrace(); }finally { DBUtil.closeAll(); } return doctors; }
时间: 2024-01-23 22:02:00 浏览: 130
这段代码看起来像是一个Java函数,它实现了从数据库中查询医生信息的功能。其中,where参数表示查询条件,o参数是查询条件中需要用到的参数数组。该函数返回一个List类型的对象,里面存放了查询得到的所有医生信息。
在函数体内,首先创建了一个空的List对象,用于存放查询得到的医生信息。然后,根据传入的查询条件和参数,构造了一个SQL语句,并调用DBUtil的executeQuery方法执行该语句,得到一个ResultSet类型的结果集。
接着,通过遍历ResultSet中的数据,将每个医生的信息存入一个Doctor对象中,并加入到之前创建的List对象中。最后,关闭数据库连接,返回包含所有医生信息的List对象。
相关问题
QueryWrapper<ChAppDoctor> queryWrapper = new QueryWrapper<>();模糊查询
QueryWrapper是MyBatis-Plus中常用的一种动态SQL构建工具,用于构造复杂的SQL查询条件。在这个例子中,`QueryWrapper<ChAppDoctor>`创建了一个针对`ChAppDoctor`实体类的对象,它是一个泛型包装器,用于对数据库表中的数据进行模糊查询。
当你使用`new QueryWrapper<>()`初始化一个空的QueryWrapper时,你可以通过一系列方法链式调用来添加查询条件,例如`like`, `eq`, `gt`等,它们分别对应于SQL中的LIKE、等于和大于操作符。例如:
```java
queryWrapper.like("name", "%关键字%"); // 模糊查询name字段包含关键字
queryWrapper.eq("status", 1); // 精确查询status字段等于1
// 全部组合起来
List<ChAppDoctor> doctors = chAppDoctorMapper.selectList(queryWrapper);
```
这将返回所有`ChAppDoctor`表中`name`字段包含指定关键词或者`status`字段等于1的数据。
Consider the following information about the pharmacies, patients and drugs: ● (1) Patients are identified by an SSN, and their names, addresses, and ages must be recorded. ● (2) Doctors are identified by an SSN. For each doctor, the name, specialty, and years of experience must be recorded. ● (3) Each pharmaceutical company (制药公司) is identified by name and has a phone number. ● (4) For each drug, the trade name and formula(成份)must be recorded. Each drug is produced by a given pharmaceutical company, and the trade name identifies a drug uniquely from among the products of that company. ● (5) Each pharmacy(药房) has a name, address, and phone number. Each pharmacy is identified by ID. ●(6) Every patient has a primary doctor. Every doctor has at least one patient. ● (7) Each pharmacy sells several drugs and has a price for each. A drug could be sold at several pharmacies, and the price could vary from one pharmacy to another. ● (8) Doctors prescribe drugs for patients. A doctor could prescribe one or more drugs for several patients, and a patient could obtain prescriptions from several doctors. Each prescription has a date and a quantity associated with it. ●(9) Pharmaceutical companies have long term contracts with pharmacies. A pharmaceutical company can contract with several pharmacies, and a pharmacy can contract with several pharmaceutical companies. For each contract, you have to store a start date, an end date. 用ER图进行描述用python代码表示
根据上述信息,可以设计以下实体和关系:
实体:
- Patient (SSN, Name, Address, Age)
- Doctor (SSN, Name, Specialty, Years of Experience)
- Pharmaceutical Company (Name, Phone Number)
- Drug (Trade Name, Formula)
- Pharmacy (ID, Name, Address, Phone Number)
关系:
- Produces (Pharmaceutical Company, Trade Name)
- Sells (Pharmacy, Trade Name, Price)
- Prescribes (Doctor, SSN, Trade Name, Patient, SSN, Date, Quantity)
- Contracts (Pharmaceutical Company, Pharmacy, Start Date, End Date)
- Has Primary Doctor (Patient, SSN, Doctor, SSN)
以下是用Python代码表示的实体和关系:
```python
# 实体类
class Patient:
def __init__(self, ssn, name, address, age):
self.ssn = ssn
self.name = name
self.address = address
self.age = age
class Doctor:
def __init__(self, ssn, name, specialty, years_of_experience):
self.ssn = ssn
self.name = name
self.specialty = specialty
self.years_of_experience = years_of_experience
class PharmaceuticalCompany:
def __init__(self, name, phone_number):
self.name = name
self.phone_number = phone_number
class Drug:
def __init__(self, trade_name, formula, pharmaceutical_company):
self.trade_name = trade_name
self.formula = formula
self.pharmaceutical_company = pharmaceutical_company
class Pharmacy:
def __init__(self, id, name, address, phone_number):
self.id = id
self.name = name
self.address = address
self.phone_number = phone_number
# 关系类
class Produces:
def __init__(self, pharmaceutical_company, drug):
self.pharmaceutical_company = pharmaceutical_company
self.drug = drug
class Sells:
def __init__(self, pharmacy, drug, price):
self.pharmacy = pharmacy
self.drug = drug
self.price = price
class Prescribes:
def __init__(self, doctor, patient, drug, date, quantity):
self.doctor = doctor
self.patient = patient
self.drug = drug
self.date = date
self.quantity = quantity
class Contracts:
def __init__(self, pharmaceutical_company, pharmacy, start_date, end_date):
self.pharmaceutical_company = pharmaceutical_company
self.pharmacy = pharmacy
self.start_date = start_date
self.end_date = end_date
class HasPrimaryDoctor:
def __init__(self, patient, doctor):
self.patient = patient
self.doctor = doctor
```
注意:上述代码只是一种示例实现,具体实现方式可能会因具体情况而有所不同。
阅读全文
相关推荐
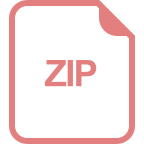
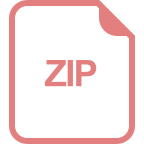
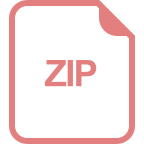
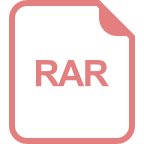
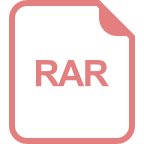
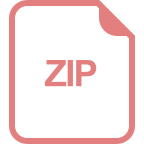
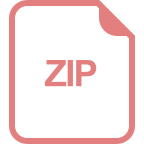
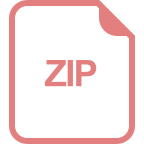
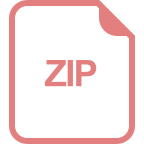
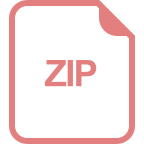
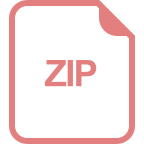
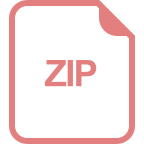
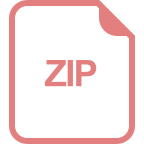
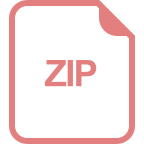
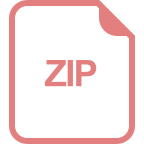
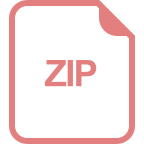