用eclipse创建jsp页面,连接mysql数据库bus,页面有两个下拉框,选择出发地和目的地并点击查询即可在当前页面以列表显示车次信息,显示车次信息的后面有删除和修改按钮代码
时间: 2024-03-12 10:45:56 浏览: 105
好的,以下是使用 Eclipse 创建 JSP 页面连接 MySQL 数据库 bus,实现选择出发地和目的地并查询车次信息的代码:
1. 首先在 Eclipse 中创建一个 Dynamic Web Project,命名为 BusSystem。
2. 在项目中创建一个名为 index.jsp 的 JSP 页面,在页面中编写以下代码:
```
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>公交车查询系统</title>
</head>
<body>
<h1>公交车查询系统</h1>
<form action="busList.jsp" method="post">
<label>出发地:</label>
<select name="start">
<option value="北京">北京</option>
<option value="上海">上海</option>
<option value="广州">广州</option>
<option value="深圳">深圳</option>
</select>
<label>目的地:</label>
<select name="end">
<option value="北京">北京</option>
<option value="上海">上海</option>
<option value="广州">广州</option>
<option value="深圳">深圳</option>
</select>
<input type="submit" value="查询">
</form>
</body>
</html>
```
3. 在项目中创建一个名为 busList.jsp 的 JSP 页面,在页面中编写以下代码:
```
<%@ page import="java.sql.*" %>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>车次信息列表</title>
</head>
<body>
<h1>车次信息列表</h1>
<table border="1">
<tr>
<th>编号</th>
<th>车次</th>
<th>出发地</th>
<th>目的地</th>
<th>操作</th>
</tr>
<%
String start = request.getParameter("start");
String end = request.getParameter("end");
Class.forName("com.mysql.jdbc.Driver");
Connection conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/bus", "root", "root");
Statement stmt = conn.createStatement();
String sql = "select * from bus_info where start='" + start + "' and end='" + end + "'";
ResultSet rs = stmt.executeQuery(sql);
while(rs.next()) {
int id = rs.getInt("id");
String code = rs.getString("code");
String s = rs.getString("start");
String e = rs.getString("end");
%>
<tr>
<td><%=id%></td>
<td><%=code%></td>
<td><%=s%></td>
<td><%=e%></td>
<td>
<a href="delete.jsp?id=<%=id%>">删除</a>
<a href="edit.jsp?id=<%=id%>">修改</a>
</td>
</tr>
<%
}
rs.close();
stmt.close();
conn.close();
%>
</table>
</body>
</html>
```
4. 在项目中创建一个名为 delete.jsp 的 JSP 页面,在页面中编写以下代码:
```
<%@ page import="java.sql.*" %>
<%
int id = Integer.parseInt(request.getParameter("id"));
Class.forName("com.mysql.jdbc.Driver");
Connection conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/bus", "root", "root");
Statement stmt = conn.createStatement();
String sql = "delete from bus_info where id=" + id;
stmt.executeUpdate(sql);
stmt.close();
conn.close();
response.sendRedirect("busList.jsp");
%>
```
5. 在项目中创建一个名为 edit.jsp 的 JSP 页面,在页面中编写以下代码:
```
<%@ page import="java.sql.*" %>
<%
int id = Integer.parseInt(request.getParameter("id"));
Class.forName("com.mysql.jdbc.Driver");
Connection conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/bus", "root", "root");
Statement stmt = conn.createStatement();
String sql = "select * from bus_info where id=" + id;
ResultSet rs = stmt.executeQuery(sql);
rs.next();
String code = rs.getString("code");
String start = rs.getString("start");
String end = rs.getString("end");
rs.close();
stmt.close();
conn.close();
%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>修改车次信息</title>
</head>
<body>
<h1>修改车次信息</h1>
<form action="update.jsp" method="post">
<input type="hidden" name="id" value="<%=id%>">
<label>车次:</label>
<input type="text" name="code" value="<%=code%>"><br>
<label>出发地:</label>
<select name="start">
<option value="北京" <%=start.equals("北京")?"selected":""%>>北京</option>
<option value="上海" <%=start.equals("上海")?"selected":""%>>上海</option>
<option value="广州" <%=start.equals("广州")?"selected":""%>>广州</option>
<option value="深圳" <%=start.equals("深圳")?"selected":""%>>深圳</option>
</select><br>
<label>目的地:</label>
<select name="end">
<option value="北京" <%=end.equals("北京")?"selected":""%>>北京</option>
<option value="上海" <%=end.equals("上海")?"selected":""%>>上海</option>
<option value="广州" <%=end.equals("广州")?"selected":""%>>广州</option>
<option value="深圳" <%=end.equals("深圳")?"selected":""%>>深圳</option>
</select><br>
<input type="submit" value="修改">
</form>
</body>
</html>
```
6. 在项目中创建一个名为 update.jsp 的 JSP 页面,在页面中编写以下代码:
```
<%@ page import="java.sql.*" %>
<%
int id = Integer.parseInt(request.getParameter("id"));
String code = request.getParameter("code");
String start = request.getParameter("start");
String end = request.getParameter("end");
Class.forName("com.mysql.jdbc.Driver");
Connection conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/bus", "root", "root");
Statement stmt = conn.createStatement();
String sql = "update bus_info set code='" + code + "',start='" + start + "',end='" + end + "' where id=" + id;
stmt.executeUpdate(sql);
stmt.close();
conn.close();
response.sendRedirect("busList.jsp");
%>
```
通过以上代码,可以实现选择出发地和目的地并查询车次信息的功能,并在车次信息列表中显示车次信息,同时提供删除和修改车次信息的功能。
阅读全文
相关推荐





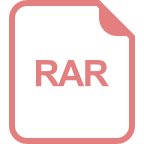








