mybatis分页的方式
时间: 2023-10-31 17:46:27 浏览: 88
Mybatis分页有两种方式:一种是采用Mybatis官方提供的分页插件PageHelper,另一种是手写分页SQL语句。使用PageHelper插件可以方便地实现分页功能,只需要在查询语句中添加对应的分页参数即可;手写分页SQL语句则需要自己编写相关SQL语句。两种方式都有各自的优缺点,具体使用哪种方式需要根据具体情况选择。
相关问题
mybatis分页插件_springBoot中两种配置mybatis分页插件方式
在 Spring Boot 中,可以使用两种方式配置 MyBatis 分页插件:
1. 使用 PageHelper 自动配置
PageHelper 是一个开源的 MyBatis 分页插件,支持多种数据库,使用起来非常方便。在 Spring Boot 中,可以使用 PageHelper 的自动配置功能,只需要在 pom.xml 中引入 PageHelper 依赖,然后在 application.properties 或 application.yml 中添加配置即可。
例如,在 pom.xml 中添加如下依赖:
```xml
<dependency>
<groupId>com.github.pagehelper</groupId>
<artifactId>pagehelper-spring-boot-starter</artifactId>
<version>1.2.13</version>
</dependency>
```
然后在 application.properties 或 application.yml 中添加如下配置:
```properties
# 开启分页插件
pagehelper.helperDialect=mysql
pagehelper.reasonable=true
pagehelper.supportMethodsArguments=true
pagehelper.params=count=countSql
```
2. 使用 MyBatis-Plus
MyBatis-Plus 是一个开源的 MyBatis 增强工具包,其中包含了分页插件。在 Spring Boot 中,可以使用 MyBatis-Plus 的自动配置功能,只需要在 pom.xml 中引入 MyBatis-Plus 依赖,然后在 application.properties 或 application.yml 中添加配置即可。
例如,在 pom.xml 中添加如下依赖:
```xml
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-boot-starter</artifactId>
<version>3.1.2</version>
</dependency>
```
然后在 application.properties 或 application.yml 中添加如下配置:
```properties
# 开启分页插件
mybatis-plus.configuration.properties.pagehelper.helperDialect=mysql
```
mybatis分页 mybatis- plus分页
MyBatis是一个优秀的持久层框架,而MyBatis-Plus是在MyBatis基础上进行了扩展和增强的框架。它们都提供了分页功能来方便处理大量数据的查询操作。
在MyBatis中实现分页可以通过使用插件或者自定义SQL语句来实现,而MyBatis-Plus分页,你可以按照以下步骤进行操作:
1. 在你的实体类中添加分页参数,例如`current`表示当前页码,`size`表示每页显示的记录数。
2. 在你的Mapper接口中继承`BaseMapper`接口,该接口提供了一些常用的CRUD方法,包括分页查询方法。
3. 在你的Service层中调用分页查询方法,传入分页参数和查询条件。
4. 在Controller层中接收分页结果,并返回给前端展示。
下面是一个示例代码:
```java
// 实体类
public class User {
private Long id;
private String name;
// 省略getter和setter方法
}
// Mapper接口
public interface UserMapper extends BaseMapper<User> {
// 省略其他方法
}
// Service层
@Service
public class UserService {
@Autowired
private UserMapper userMapper;
public IPage<User> getUserList(int current, int size) {
Page<User> page = new Page<>(current, size);
QueryWrapper<User> queryWrapper = new QueryWrapper<>();
// 设置查询条件
// queryWrapper.eq("name", "张三");
return userMapper.selectPage(page, queryWrapper);
}
}
// Controller层
@RestController
public class UserController {
@Autowired
private UserService userService;
@GetMapping("/users")
public IPage<User> getUserList(@RequestParam(defaultValue = "1") int current,
@RequestParam(defaultValue = "10") int size) {
return userService.getUserList(current, size);
}
}
```
以上示例中,`getUserList`方法实现了分页查询功能,通过调用`selectPage`方法实现分页查询,并将查询结果封装到`IPage`对象中返回给前端。
阅读全文
相关推荐
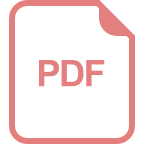
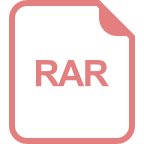
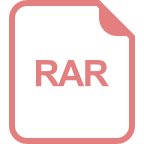
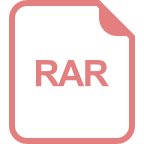
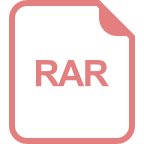
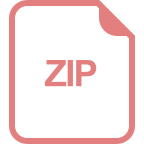




