Write a C program that asks to the user 20 heights of persons as integer values in centimeter. The program should then print the (integer) average height of the persons. If any of the values is greater than 240 or smaller than 50, the program should print the message “Check the inputs.” before ending.
时间: 2024-04-08 16:35:37 浏览: 15
Certainly! Here's the C program that meets your requirements:
```c
#include <stdio.h>
int main() {
int heights[20];
int i, sum = 0;
int average;
printf("Enter the heights of 20 persons (in centimeters):\n");
for (i = 0; i < 20; i++) {
printf("Enter the height of person %d: ", i + 1);
scanf("%d", &heights[i]);
if (heights[i] > 240 || heights[i] < 50) {
printf("Check the inputs.\n");
return 0;
}
sum += heights[i];
}
average = sum / 20;
printf("The average height is: %d cm\n", average);
return 0;
}
```
In this program, an array `heights` is used to store the user input values for heights. The program asks the user to enter the height of each person, one by one. If any height value is greater than 240 or smaller than 50, it will print the message "Check the inputs" and exit the program.
After getting all the heights, it calculates the sum of all the heights and then divides it by the number of persons (20) to get the average height. Finally, it prints the average height in centimeters as an integer value.
相关推荐
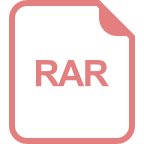
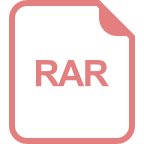
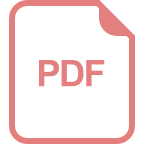














