d2i_PublicKey接口需要传入的公钥数据格式应该是怎么样的, 我应该怎么从一个pem证书里面提取该数据。请使用linux c语言实现
时间: 2024-03-17 20:43:51 浏览: 157
d2i_PublicKey接口需要传入DER编码格式的公钥数据。如果你有一个PEM格式的证书文件,可以使用OpenSSL库中的函数来提取DER编码格式的公钥数据。下面是一个简单的C代码片段,它使用OpenSSL库中的函数从PEM证书文件中提取公钥数据并将其转换为DER编码格式:
```c
#include <stdio.h>
#include <openssl/pem.h>
#include <openssl/evp.h>
int main()
{
FILE *fp;
EVP_PKEY *pubkey;
X509 *cert;
const unsigned char *p_der;
int len;
// 打开PEM证书文件
fp = fopen("cert.pem", "r");
if (fp == NULL) {
printf("Failed to open certificate file\n");
return 1;
}
// 读取PEM证书
cert = PEM_read_X509(fp, NULL, NULL, NULL);
if (cert == NULL) {
printf("Failed to read certificate\n");
fclose(fp);
return 1;
}
// 从证书中提取公钥
pubkey = X509_get_pubkey(cert);
if (pubkey == NULL) {
printf("Failed to extract public key from certificate\n");
fclose(fp);
return 1;
}
// 将公钥转换为DER编码格式
len = i2d_PublicKey(pubkey, NULL);
p_der = malloc(len);
if (!p_der) {
printf("Memory allocation failed\n");
fclose(fp);
return 1;
}
i2d_PublicKey(pubkey, &p_der);
// 输出DER编码格式的公钥数据
printf("DER-encoded public key:\n");
for (int i = 0; i < len; i++) {
printf("%02x", p_der[i]);
}
printf("\n");
// 释放资源
free(p_der);
EVP_PKEY_free(pubkey);
X509_free(cert);
fclose(fp);
return 0;
}
```
这个程序假定PEM证书文件名为"cert.pem",并且使用了OpenSSL库中的PEM_read_X509和i2d_PublicKey函数来读取和处理证书。运行这个程序后,它将输出DER编码格式的公钥数据。
阅读全文
相关推荐










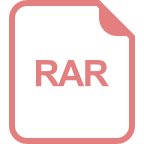






