c++设计小型企业的员工主要有 4 类:经理、技术员、销售员和计时工。要求存 储员工的工号、姓名、岗位、月工资等基本信息
时间: 2023-10-20 09:08:57 浏览: 185
可以使用面向对象的思想设计一个Employee类,然后派生出经理、技术员、销售员和计时工等子类。每个子类中可以添加特定的属性和方法。例如,经理可能有一个部门属性和管理下属员工的方法,销售员可能有一个销售额属性和计算提成的方法。
以下是一个简单的示例代码:
```c++
#include <string>
#include <iostream>
using namespace std;
class Employee {
protected:
int id;
string name;
string position;
float salary;
public:
Employee(int _id, string _name, string _position, float _salary) :
id(_id), name(_name), position(_position), salary(_salary) {}
virtual void display() {
cout << "ID: " << id << endl;
cout << "Name: " << name << endl;
cout << "Position: " << position << endl;
cout << "Salary: " << salary << endl;
}
};
class Manager : public Employee {
private:
string department;
public:
Manager(int _id, string _name, string _department, float _salary) :
Employee(_id, _name, "Manager", _salary), department(_department) {}
void manage() {
cout << "Managing department " << department << endl;
}
void display() {
Employee::display();
cout << "Department: " << department << endl;
}
};
class Technician : public Employee {
private:
string skill;
public:
Technician(int _id, string _name, string _skill, float _salary) :
Employee(_id, _name, "Technician", _salary), skill(_skill) {}
void fix() {
cout << "Fixing with " << skill << endl;
}
void display() {
Employee::display();
cout << "Skill: " << skill << endl;
}
};
class Salesman : public Employee {
private:
float sales;
public:
Salesman(int _id, string _name, float _sales, float _salary) :
Employee(_id, _name, "Salesman", _salary), sales(_sales) {}
float getCommission() {
return sales * 0.1; // 假设提成为销售额的 10%
}
void display() {
Employee::display();
cout << "Sales: " << sales << endl;
}
};
class HourlyWorker : public Employee {
private:
float hours;
public:
HourlyWorker(int _id, string _name, float _hours, float _salary) :
Employee(_id, _name, "Hourly Worker", _salary), hours(_hours) {}
float getPay() {
return hours * salary; // 小时工的工资为时薪乘以工作时间
}
void display() {
Employee::display();
cout << "Hours: " << hours << endl;
}
};
int main() {
Employee* e1 = new Manager(1, "John", "Sales", 5000);
Employee* e2 = new Technician(2, "Mary", "Programming", 4000);
Employee* e3 = new Salesman(3, "Tom", 10000, 3000);
Employee* e4 = new HourlyWorker(4, "Alice", 80, 50);
e1->display();
e2->display();
e3->display();
e4->display();
delete e1;
delete e2;
delete e3;
delete e4;
return 0;
}
```
在这个示例中,我们使用了虚函数和基类指针来实现多态。通过基类指针可以方便地调用子类的方法,而且还可以将不同类型的员工对象存储在同一个数组或容器中,实现更加灵活的管理。
阅读全文
相关推荐

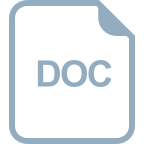
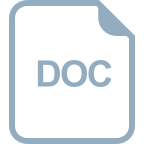












