springboot websocket客户端
时间: 2023-09-05 13:01:37 浏览: 215
### 回答1:
Spring Boot WebSocket客户端是一个用于与WebSocket服务器进行通信的Java客户端。它提供了一个简单的API,使得开发人员可以轻松地创建WebSocket连接并发送和接收消息。Spring Boot WebSocket客户端还支持STOMP协议,这是一种基于消息的协议,用于在客户端和服务器之间进行双向通信。使用Spring Boot WebSocket客户端,开发人员可以轻松地构建实时Web应用程序和在线游戏等应用程序。
### 回答2:
Spring Boot提供了对WebSocket的支持,可以很方便地创建WebSocket客户端。
首先,在pom.xml文件中添加以下依赖:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-websocket</artifactId>
</dependency>
```
接下来,在项目的配置类上加上@EnableWebSocketMessageBroker注解,启用Spring的WebSocket消息代理功能。
然后,创建一个WebSocket客户端类,可以继承WebSocketClient或实现ClientWebSocket类。在这个类中,我们可以重写一些方法,例如连接建立、关闭、接收消息等。
下面是一个简单的WebSocket客户端类示例:
```java
import org.springframework.messaging.converter.StringMessageConverter;
import org.springframework.messaging.simp.stomp.StompHeaders;
import org.springframework.messaging.simp.stomp.StompSessionHandler;
import org.springframework.messaging.simp.stomp.StompSessionHandlerAdapter;
import org.springframework.web.socket.client.WebSocketClient;
import org.springframework.web.socket.client.standard.StandardWebSocketClient;
import org.springframework.web.socket.messaging.WebSocketStompClient;
import java.util.concurrent.ExecutionException;
import java.util.concurrent.TimeUnit;
import java.util.concurrent.TimeoutException;
public class MyWebSocketClient {
public static void main(String[] args) {
WebSocketClient client = new StandardWebSocketClient();
WebSocketStompClient stompClient = new WebSocketStompClient(client);
stompClient.setMessageConverter(new StringMessageConverter());
String url = "ws://localhost:8080/ws"; // WebSocket服务器地址
StompSessionHandler sessionHandler = new MyStompSessionHandler(); // 自定义处理器
try {
stompClient.connect(url, sessionHandler).get(10, TimeUnit.SECONDS);
} catch (InterruptedException | ExecutionException | TimeoutException e) {
e.printStackTrace();
}
}
private static class MyStompSessionHandler extends StompSessionHandlerAdapter {
@Override
public void afterConnected(StompSession session, StompHeaders connectedHeaders) {
session.subscribe("/topic/messages", this); // 订阅主题
session.send("/app/sendMessage", "Hello, WebSocket!"); // 发送消息
}
@Override
public void handleFrame(StompHeaders headers, Object payload) {
System.out.println("Received message: " + payload); // 收到消息的处理逻辑
}
}
}
```
在这个例子中,我们创建了一个WebSocketStompClient对象,并设置使用StringMessageConverter转换消息。然后,我们创建一个MyStompSessionHandler对象,继承自StompSessionHandlerAdapter,并重写了afterConnected方法和handleFrame方法,用于处理连接建立后的逻辑和接收到消息的逻辑。
最后,我们使用WebSocketStompClient的connect方法连接到指定的WebSocket服务器地址,并传入我们创建的处理器对象。
这样,就可以通过Spring Boot创建WebSocket客户端了。
阅读全文
相关推荐
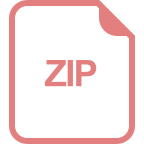







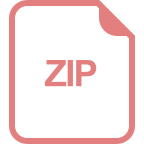
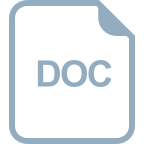




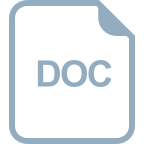

