python通过读取txt文件中的数字,绘制数字三角形的图(每个数字作为节点,每个节点用线段链接),并使用Q-learning算法寻找使最佳路径使该路径上的数字加和最大并绘制最佳路径图
时间: 2024-05-15 20:19:54 浏览: 70
对于第一部分,读取txt文件中的数字并绘制数字三角形的图,可以使用Python中的matplotlib库来实现。具体实现步骤如下:
1. 读取txt文件中的数字,存储到列表中。
2. 根据列表中的数字,构建数字三角形。
3. 使用matplotlib库中的plot函数,绘制数字三角形。
下面是一个简单的实现代码:
```python
import matplotlib.pyplot as plt
# 读取txt文件中的数字
with open('triangle.txt', 'r') as f:
lines = f.readlines()
nums = []
for line in lines:
nums.append(list(map(int, line.strip().split())))
# 构建数字三角形
n = len(nums)
for i in range(n-2, -1, -1):
for j in range(len(nums[i])):
nums[i][j] += max(nums[i+1][j], nums[i+1][j+1])
# 绘制数字三角形
fig, ax = plt.subplots()
for i in range(n):
for j in range(len(nums[i])):
ax.plot(j, n-i-1, 'o', markersize=10, color='blue')
ax.text(j, n-i-1, str(nums[i][j]), ha='center', va='center')
if i < n-1:
ax.plot([j, j+1], [n-i-1, n-i-2], color='black')
plt.show()
```
对于第二部分,使用Q-learning算法寻找使最佳路径使该路径上的数字加和最大并绘制最佳路径图,可以使用以下步骤:
1. 定义状态:数字三角形中每个数字的位置。
2. 定义动作:从当前位置向左下或右下移动一步。
3. 定义奖励:到达数字三角形底部时,奖励为最终路径上的数字加和。
4. 使用Q-learning算法进行训练,更新状态-动作值函数。
5. 根据训练后的状态-动作值函数,选择最佳路径并绘制路径图。
下面是一个简单的实现代码:
```python
import numpy as np
import matplotlib.pyplot as plt
# 读取txt文件中的数字
with open('triangle.txt', 'r') as f:
lines = f.readlines()
nums = []
for line in lines:
nums.append(list(map(int, line.strip().split())))
# 定义状态和动作
states = [(i, j) for i in range(len(nums)) for j in range(len(nums[i]))]
actions = [(1, 1), (1, 0)]
# 定义奖励
def reward(state):
i, j = state
if i == len(nums) - 1:
return nums[i][j]
else:
return 0
# 初始化Q值为0
Q = {}
for state in states:
for action in actions:
Q[(state, action)] = 0
# 定义Q-learning算法
def q_learning(alpha, gamma, epsilon, num_episodes):
global Q
rewards = []
for episode in range(num_episodes):
state = (0, 0)
total_reward = 0
while state[0] < len(nums) - 1:
# 选择动作
if np.random.uniform(0, 1) < epsilon:
action = actions[np.random.randint(0, 2)]
else:
q_values = [Q[(state, a)] for a in actions]
max_q = max(q_values)
if q_values.count(max_q) > 1:
best_actions = [a for a in actions if Q[(state, a)] == max_q]
action = best_actions[np.random.randint(0, len(best_actions))]
else:
action = actions[np.argmax(q_values)]
# 执行动作,更新状态
next_state = (state[0] + action[0], state[1] + action[1])
reward_value = reward(next_state)
total_reward += reward_value
if next_state[0] < len(nums) - 1:
next_q_values = [Q[(next_state, a)] for a in actions]
max_next_q = max(next_q_values)
else:
max_next_q = 0
# 更新Q值
Q[(state, action)] += alpha * (reward_value + gamma * max_next_q - Q[(state, action)])
state = next_state
rewards.append(total_reward)
return rewards
# 训练Q-learning算法
alpha = 0.5
gamma = 0.9
epsilon = 0.1
num_episodes = 1000
rewards = q_learning(alpha, gamma, epsilon, num_episodes)
# 选择最佳路径
state = (0, 0)
path = [(0, 0)]
while state[0] < len(nums) - 1:
q_values = [Q[(state, a)] for a in actions]
max_q = max(q_values)
if q_values.count(max_q) > 1:
best_actions = [a for a in actions if Q[(state, a)] == max_q]
action = best_actions[np.random.randint(0, len(best_actions))]
else:
action = actions[np.argmax(q_values)]
state = (state[0] + action[0], state[1] + action[1])
path.append(state)
# 绘制数字三角形和最佳路径
fig, ax = plt.subplots()
for i in range(len(nums)):
for j in range(len(nums[i])):
ax.plot(j, len(nums)-i-1, 'o', markersize=10, color='blue')
ax.text(j, len(nums)-i-1, str(nums[i][j]), ha='center', va='center')
if i < len(nums) - 1:
ax.plot([j, j+1], [len(nums)-i-1, len(nums)-i-2], color='black')
for i in range(len(path)-1):
ax.plot([path[i][1], path[i+1][1]], [len(nums)-path[i][0]-1, len(nums)-path[i+1][0]-1], color='red', linewidth=2)
plt.show()
```
阅读全文
相关推荐

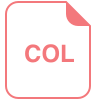
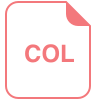
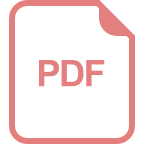
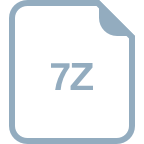
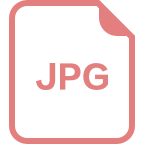
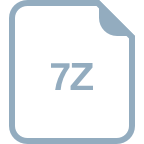
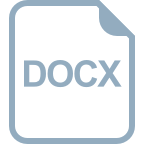