springboot文件上传
时间: 2023-11-05 12:02:28 浏览: 42
Spring Boot 提供了一个简单的方式来处理文件上传,通过使用 Spring Boot 的自动配置可以轻松地实现文件上传功能。
1. 配置文件上传
在 Spring Boot 中,我们可以使用 MultipartConfigElement 类来配置文件上传。可以通过在 application.properties 或 application.yml 中添加如下配置:
application.properties
```
# 文件上传配置
spring.servlet.multipart.enabled=true
spring.servlet.multipart.max-file-size=10MB
spring.servlet.multipart.max-request-size=100MB
spring.servlet.multipart.file-size-threshold=0
```
application.yml
```
# 文件上传配置
spring:
servlet:
multipart:
enabled: true
max-file-size: 10MB
max-request-size: 100MB
file-size-threshold: 0
```
- `spring.servlet.multipart.enabled`:是否启用文件上传,默认为 true。
- `spring.servlet.multipart.max-file-size`:允许上传文件的最大大小,默认为 1MB。
- `spring.servlet.multipart.max-request-size`:允许上传请求的最大大小,默认为 10MB。
- `spring.servlet.multipart.file-size-threshold`:设置一个临界值,当上传文件的大小小于该临界值时,文件将被保存在内存中,否则将被保存在磁盘上。
2. 实现文件上传控制器
在 Spring Boot 中,可以使用 Spring MVC 来处理文件上传请求。我们可以通过添加 `@RestController` 注解来创建一个 RESTful 风格的控制器,并使用 `@PostMapping` 注解来处理文件上传请求。
```java
@RestController
public class FileUploadController {
@PostMapping("/upload")
public String handleFileUpload(@RequestParam("file") MultipartFile file) {
try {
String fileName = file.getOriginalFilename();
byte[] bytes = file.getBytes();
// 处理文件上传逻辑
return "上传成功!";
} catch (IOException e) {
e.printStackTrace();
return "上传失败!";
}
}
}
```
在上面的代码中,我们定义了一个 `handleFileUpload()` 方法,该方法使用 `@RequestParam` 注解来接收文件上传请求中的 `file` 参数,并将上传的文件保存到服务器中。
3. 创建文件上传页面
在前端页面中,我们可以使用 HTML 表单来实现文件上传功能。下面是一个简单的文件上传表单示例:
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>文件上传示例</title>
</head>
<body>
<form action="/upload" method="post" enctype="multipart/form-data">
<input type="file" name="file" />
<button type="submit">上传</button>
</form>
</body>
</html>
```
在上面的代码中,我们使用了 `enctype="multipart/form-data"` 属性来告诉浏览器,这是一个文件上传表单。
4. 测试文件上传
完成以上步骤后,我们可以启动 Spring Boot 项目并访问文件上传页面,选择一个文件并点击上传按钮,即可上传文件到服务器中。
上传成功后,我们可以在控制台中看到上传成功的提示信息。
参考资料:
- [Spring Boot 文件上传](https://www.yiibai.com/spring-boot/spring_boot_file_upload.html)
- [Spring Boot 官方文档:Uploading Files](https://docs.spring.io/spring-boot/docs/current/reference/html/spring-boot-features.html#boot-features-file-upload)
相关推荐










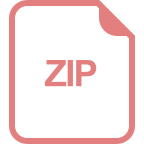