angular在父组件上触发子组件方法
时间: 2023-08-25 18:07:21 浏览: 55
### 回答1:
要在父组件中触发子组件的方法,可以使用 Angular 的 ViewChild 装饰器和 ngAfterViewInit 钩子函数。
首先,在父组件的模板中使用子组件的选择器标记子组件:
```
<app-child #childComponent></app-child>
```
然后,在父组件的代码中使用 ViewChild 装饰器获取子组件的引用:
```
import { Component, ViewChild } from '@angular/core';
import { ChildComponent } from './child.component';
@Component({
selector: 'app-parent',
template: `
<app-child #childComponent></app-child>
<button (click)="callChildMethod()">Call Child Method</button>
`
})
export class ParentComponent {
@ViewChild('childComponent') childComponent: ChildComponent;
callChildMethod() {
this.childComponent.childMethod();
}
}
```
最后,在 ngAfterViewInit 钩子函数中调用子组件的方法:
```
import { Component, ViewChild, AfterViewInit } from '@angular/core';
import { ChildComponent } from './child.component';
@Component({
selector: 'app-parent',
template: `
<app-child #childComponent></app-child>
<button (click)="callChildMethod()">Call Child Method</button>
`
})
export class ParentComponent implements AfterViewInit {
@ViewChild('childComponent') childComponent: ChildComponent;
ngAfterViewInit() {
this.childComponent.childMethod();
}
}
```
这样,当父组件的按钮被点击或者父组件初始化完成后,都可以触发子组件的方法。
### 回答2:
在Angular中,父组件可以通过ViewChild装饰器来访问子组件的方法。以下是一个简单的示例:
在父组件的HTML模板中,我们需要使用子组件的选择器来承载子组件:
```
<app-child-component></app-child-component>
```
然后,在父组件的Typescript文件中,我们可以使用ViewChild装饰器来获得对子组件实例的引用,从而触发子组件的方法。假设子组件有一个名为"childMethod"的方法:
```
import { Component, ViewChild } from '@angular/core';
import { ChildComponent } from './child-component.component';
@Component({
selector: 'app-parent-component',
template: '...'
})
export class ParentComponent {
@ViewChild(ChildComponent) childComponent: ChildComponent;
triggerChildMethod() {
this.childComponent.childMethod();
}
}
```
在上面的例子中,我们通过ViewChild装饰器将ChildComponent的实例赋值给了childComponent属性。然后,我们可以在triggerChildMethod方法中调用childComponent的childMethod。
通过以上步骤,父组件就可以触发子组件的方法了。可以在父组件中的任何方法或事件中调用triggerChildMethod来执行子组件中的方法。
需要注意的是,ViewChild装饰器可以接受一个字符串参数,用于指定子组件的选择器,以便在多个子组件中选择需要引用的组件实例。
### 回答3:
在Angular中,父组件可以通过ViewChild或ViewChildren装饰器来获取子组件的实例,并调用子组件的方法。
首先,在父组件中引入ViewChild装饰器,并使用它来声明一个变量以获取子组件的实例。例如:
```typescript
import { Component, ViewChild } from '@angular/core';
import { ChildComponent } from './child.component';
@Component({
selector: 'parent-component',
template: `
<child-component></child-component>
`
})
export class ParentComponent {
@ViewChild(ChildComponent) childComponent: ChildComponent;
callChildMethod() {
this.childComponent.childMethod();
}
}
```
在上述示例中,我们通过ViewChild装饰器创建了一个名为childComponent的变量,类型为ChildComponent,用于获取子组件的实例。
接下来,我们可以在父组件的方法中调用子组件的方法。在上述示例中,我们创建了一个名为callChildMethod的方法,通过this.childComponent.childMethod()调用了子组件的childMethod方法。
需要注意的是,调用子组件的方法需要确保在子组件已被加载之后进行。可以在父组件的生命周期钩子函数ngAfterViewInit中进行调用,以确保子组件已完全加载。
总结起来,父组件可以通过ViewChild装饰器获取子组件的实例,然后调用子组件的方法来触发子组件的操作。
相关推荐
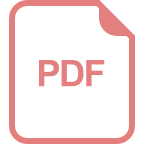
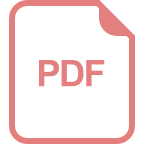
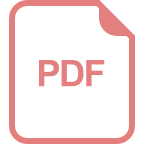














