python编程4)根据用户输入的 算天数。 编写函数 isLeap ( year ),用于判断 year 是不是闰年,若是闰年则返回 True ,否则返回 False 。判断闰年的依据是: year 为非整百年且为4的倍数是闰年,为整百年且为400的倍数是闰年。例如:2019年不是闰年,2020年是闰年,2100年不是闰年,2000年是闰年。 编写函数 days ( year , month ),用于计算 year 所在的 month 的天数。 days ( year , month )函数需要调用 isLeap (函数以帮助判断2月份的天数( year 若不是闰年,返回28,否则返回29)。要求程序能根据用户一次性输入的年/月/日(如2019/3/18)输入计算该日期是这一年的第几天。
时间: 2023-12-06 16:43:04 浏览: 112
可以按照如下方式实现:
```python
def isLeap(year):
if year % 4 == 0 and (year % 100 != 0 or year % 400 == 0):
return True
else:
return False
def days(year, month):
if month in (1, 3, 5, 7, 8, 10, 12):
return 31
elif month == 2:
if isLeap(year):
return 29
else:
return 28
else:
return 30
def dayOfYear(year, month, day):
total_days = 0
for i in range(1, month):
total_days += days(year, i)
total_days += day
return total_days
date_input = input("请输入日期(格式为yyyy/mm/dd):")
year, month, day = map(int, date_input.split('/'))
print("该日期是这一年的第 %d 天。" % dayOfYear(year, month, day))
```
其中,`isLeap(year)` 函数用于判断 `year` 是否为闰年,`days(year, month)` 函数用于计算 `year` 年 `month` 月的天数。在 `dayOfYear(year, month, day)` 函数中,通过循环遍历到 `month` 之前的所有月份,累加每个月的天数,再加上 `day` 就是该日期是这一年的第几天。最后,根据用户输入的日期,调用 `dayOfYear(year, month, day)` 函数即可得到该日期是这一年的第几天。
阅读全文
相关推荐
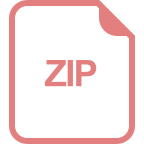
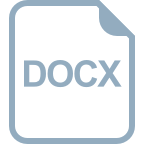















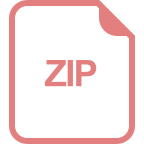