建立一点状要素服务图层,设计输入要素的程序代码
时间: 2023-11-12 19:04:18 浏览: 39
建立点要素服务图层可以使用Python中的geopandas库,以下是一个示例代码:
```python
import geopandas as gpd
# 创建一个空间参考系对象
crs = {'init': 'epsg:4326'}
# 创建一个空的地理数据框(GeoDataFrame)
points_gdf = gpd.GeoDataFrame(columns=['Name', 'Latitude', 'Longitude'], crs=crs)
# 添加新的点要素
new_point = gpd.GeoDataFrame({'Name': ['Point 1'], 'Latitude': [40.7128], 'Longitude': [-74.0060]}, geometry=gpd.points_from_xy([-74.0060], [40.7128]), crs=crs)
# 将新的点要素添加到空的地理数据框中
points_gdf = points_gdf.append(new_point)
# 将点要素服务图层保存为shapefile文件
points_gdf.to_file('points.shp')
```
这个示例代码首先创建了一个空的地理数据框,然后添加了一个名为“Point 1”的新点要素。最后,将点要素服务图层保存为shapefile文件。你可以根据需求修改代码,添加更多的点要素。
相关问题
用vs建立一点状要素服务图层,设计输入要素的程序
以下是使用Visual Studio建立点要素服务图层并设计输入要素的程序示例:
1. 首先,打开Visual Studio并创建一个新的C#控制台应用程序项目。
2. 在解决方案资源管理器中,右键单击项目名称并选择“添加”>“新建项”。
3. 在“添加新项”对话框中,选择“ArcGIS”>“Geoprocessing Tool”。
4. 在“新建工具”对话框中,输入工具名称并选择“点要素服务”作为工具类型。
5. 在“参数”选项卡中,添加需要的参数。例如,添加一个名为“x_coord”的Double类型参数,表示要素的X坐标;添加一个名为“y_coord”的Double类型参数,表示要素的Y坐标。
6. 在“代码”选项卡中,编写程序代码来创建点要素服务图层并添加输入要素。例如:
```csharp
using System;
using System.Collections.Generic;
using System.Text;
using ESRI.ArcGIS.Geodatabase;
using ESRI.ArcGIS.Geometry;
using ESRI.ArcGIS.Server;
using ESRI.ArcGIS.SOESupport;
namespace PointFeatureService
{
class PointFeatureService : SOE
{
private IServerObjectHelper _soHelper;
private ServerLogger _serverLog;
public PointFeatureService()
{
_serverLog = new ServerLogger();
}
public override void Init(IServerObjectHelper pSOH)
{
_soHelper = pSOH;
}
public override byte[] HandleRESTRequest(string Capabilities, string resourceName, string operationName, string operationInput, string outputFormat, string requestProperties, out string responseProperties)
{
responseProperties = null;
switch (operationName.ToLower())
{
case "addpoint":
return AddPoint(operationInput);
default:
throw new Exception("Unsupported operation.");
}
}
private byte[] AddPoint(string operationInput)
{
JsonObject input = new JsonObject(operationInput);
double xCoord = input.GetAsDouble("x_coord");
double yCoord = input.GetAsDouble("y_coord");
IPoint point = new PointClass();
point.X = xCoord;
point.Y = yCoord;
IFeatureClass featureClass = GetFeatureClass();
IFeature feature = featureClass.CreateFeature();
feature.Shape = point;
feature.Store();
JsonObject result = new JsonObject();
result.AddString("status", "success");
return Encoding.UTF8.GetBytes(result.ToJson());
}
private IFeatureClass GetFeatureClass()
{
IWorkspaceFactory workspaceFactory = new FileGDBWorkspaceFactoryClass();
IWorkspace workspace = workspaceFactory.OpenFromFile(@"C:\data.gdb", 0);
IFeatureWorkspace featureWorkspace = (IFeatureWorkspace)workspace;
return featureWorkspace.OpenFeatureClass("Points");
}
}
}
```
7. 在“生成”菜单中选择“生成解决方案”以生成程序集。
8. 在ArcGIS Server管理器中创建一个新的SOE(Server Object Extension)并将其指向程序集。
9. 在ArcMap中创建一个点要素类,并将其存储在一个文件地理数据库中。将要素类命名为“Points”。
10. 使用ArcGIS Server管理器中的“发布”向服务器发布点要素服务。在“发布服务”对话框中,选择“点要素服务”作为服务类型,选择点要素类作为数据源。
11. 使用ArcGIS Server管理器中的“测试”功能测试点要素服务。可以使用Postman或类似的工具向服务发送POST请求来添加输入要素。例如,使用以下JSON字符串向服务添加一个具有x坐标值为10和y坐标值为20的新要素:
```json
{
"x_coord": 10,
"y_coord": 20
}
```
以上就是使用Visual Studio建立点要素服务图层并设计输入要素的程序示例。
用vs建立一点状要素服务图层,设计输入要素的程序的代码
以下是用 C# 语言在 Visual Studio 中建立点要素服务图层并设计输入要素的示例代码:
```csharp
using ESRI.ArcGIS.ADF;
using ESRI.ArcGIS.Carto;
using ESRI.ArcGIS.Geodatabase;
using ESRI.ArcGIS.Geometry;
namespace CreateFeatureLayer
{
class Program
{
static void Main(string[] args)
{
// 创建工作空间
IWorkspaceFactory workspaceFactory = new FileGDBWorkspaceFactory();
IWorkspace workspace = workspaceFactory.OpenFromFile("C:/data/mydb.gdb", 0);
// 获取地图文档和图层
string mapDocumentPath = "C:/data/map.mxd";
IMapDocument mapDocument = new MapDocumentClass();
mapDocument.Open(mapDocumentPath, null);
IMap map = mapDocument.Map[0];
ILayer layer = map.get_Layer(0); // 假设第一个图层是点要素图层
// 获取要素类
IFeatureLayer featureLayer = layer as IFeatureLayer;
IFeatureClass featureClass = featureLayer.FeatureClass;
// 创建一个新要素
IFeature feature = featureClass.CreateFeature();
// 设置要素的几何形状
IPoint point = new PointClass();
point.PutCoords(-118.15, 34.05); // 设置点的坐标
feature.Shape = point;
// 设置要素的属性值
int index = featureClass.FindField("Name"); // 假设要素类有一个字段叫 Name
feature.set_Value(index, "MyPoint");
// 保存要素
feature.Store();
// 刷新地图并保存
mapDocument.Save(true, true);
mapDocument.Close();
}
}
}
```
在上面的示例代码中,我们首先创建了一个文件地理数据库工作空间,然后打开了一个地图文档并获取了第一个图层作为点要素图层。接着,我们获取了该要素图层的要素类,并创建了一个新的点要素。然后,我们设置了该要素的几何形状和属性值,并将其保存到要素类中。最后,我们刷新了地图并保存了地图文档。
相关推荐
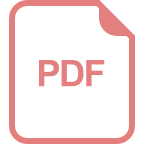
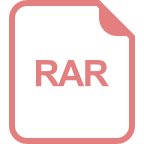
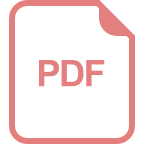












