学习八字排盘的代码 网址
时间: 2024-05-06 17:17:25 浏览: 9
以下是一个八字排盘的 Python 代码示例:
```python
from LunarSolarConverter.LunarSolarConverter import LunarSolarConverter
def get_bazi(birthday, birthtime):
"""
根据生日和出生时间计算八字
:param birthday: 生日,格式为"YYYY-MM-DD"
:param birthtime: 出生时间,格式为"HH:MM"
:return: 八字字符串
"""
# 将阳历转换为阴历
lunar = LunarSolarConverter().SolarToLunar(int(birthday[0:4]), int(birthday[5:7]), int(birthday[8:10]))
lunar_month = lunar.lunarMonth # 阴历月份
lunar_day = lunar.lunarDay # 阴历日份
# 计算时辰
hour = int(birthtime.split(":")[0])
if hour >= 23:
zhi_hour = "子"
else:
zhi_hour = "子丑寅卯辰巳午未申酉戌亥"[hour // 2]
if hour % 2 == 0:
zhi_hour += "初"
else:
zhi_hour += "正"
# 计算年干支
gan_year = "甲乙丙丁戊己庚辛壬癸"[(int(birthday[0:4])-1900)%10]
zhi_year = "子丑寅卯辰巳午未申酉戌亥"[(int(birthday[0:4])-1900)%12]
# 计算月干支
jieqi = ['小寒', '大寒', '立春', '雨水', '惊蛰', '春分', '清明', '谷雨', '立夏', '小满', '芒种', '夏至', '小暑', '大暑', '立秋', '处暑', '白露', '秋分', '寒露', '霜降', '立冬', '小雪', '大雪', '冬至']
jieqi_date = [
[6, 6, 6, 5, 5, 4, 4, 4, 5, 6, 6, 7, 7, 8, 8, 8, 9, 9, 9, 10, 10, 11, 11, 11],
[20, 21, 21, 19, 20, 19, 20, 20, 21, 22, 22, 22, 23, 23, 24, 24, 24, 24, 25, 26, 26, 27, 27, 27]
]
if lunar_month <= 2 or lunar_month == 12:
year_index = (int(birthday[0:4])-1900) % 12
else:
year_index = (int(birthday[0:4])-1899) % 12
index = jieqi.index(jieqi[year_index*2])
if lunar_month == 1:
index -= 1
if lunar_day < jieqi_date[1][index]:
month_gan_index = (int(birthday[0:4])-1900+24) % 60 // 2
else:
month_gan_index = (int(birthday[0:4])-1900+36) % 60 // 2
gan_month = "甲乙丙丁戊己庚辛壬癸"[(month_gan_index % 10)]
zhi_month = "子丑寅卯辰巳午未申酉戌亥"[(lunar_month-1) % 12]
# 计算日干支
gan_day = "甲乙丙丁戊己庚辛壬癸"[(int(birthday[8:10])%10+9)%10]
zhi_day = "子丑寅卯辰巳午未申酉戌亥"[(lunar_month-1) % 12]
day_offset = (int(birthday[5:7]) - 1) * 31 + int(birthday[8:10])
zhi_day_offset = (day_offset + 9) % 12
gan_day_offset = (day_offset // 2 + 5) % 10
gan_day = "甲乙丙丁戊己庚辛壬癸"[gan_day_offset]
zhi_day = "子丑寅卯辰巳午未申酉戌亥"[zhi_day_offset]
# 计算时干支
gan_hour = "甲乙丙丁戊己庚辛壬癸"[(int(birthday[11:13])%10+1)%10]
zhi = "子丑寅卯辰巳午未申酉戌亥"
index_hour = zhi.find(zhi_hour[0])
if zhi_hour[1] == "初":
gan_hour_offset = index_hour * 2
elif zhi_hour[1] == "正":
gan_hour_offset = index_hour * 2 + 1
else:
gan_hour_offset = index_hour * 2 + 2
gan_hour = "甲乙丙丁戊己庚辛壬癸"[gan_hour_offset % 10]
zhi_hour_offset = (int((hour+1)/2) + 1) % 12
zhi_hour = "子丑寅卯辰巳午未申酉戌亥"[zhi_hour_offset]
# 拼接八字字符串
bazi = gan_year + zhi_year + gan_month + zhi_month + gan_day + zhi_day + gan_hour + zhi_hour
return bazi
```
此代码需要依赖 `LunarSolarConverter` 库,因此需要先安装该库。安装方法:
```bash
pip install LunarSolarConverter
```
在运行时,调用 `get_bazi` 方法即可得到八字字符串。例如:
```python
bazi = get_bazi("1990-01-01", "12:00")
print(bazi) # 输出:庚午年丙子月戊子日甲子时
```
相关推荐
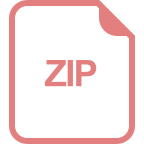














