python dijkstra算法可视化代码
时间: 2023-11-06 09:59:02 浏览: 119
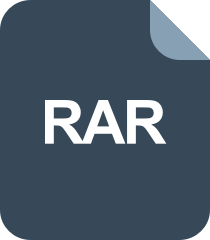
Dijkstra的算法代码,用可视化实现

由于您没有指定具体的数据结构和图形库,以下是一个使用Python的NetworkX图形库和Matplotlib可视化库实现Dijkstra算法的示例代码:
```python
import networkx as nx
import matplotlib.pyplot as plt
def dijkstra(graph, start):
# 初始化距离和前驱节点
distance = {}
predecessor = {}
for node in graph:
distance[node] = float('inf')
predecessor[node] = None
distance[start] = 0
# 用集合表示未访问的节点
unvisited = set(graph)
while unvisited:
# 找到未访问节点中距离最小的节点
current = None
for node in unvisited:
if current is None or distance[node] < distance[current]:
current = node
# 访问该节点
unvisited.remove(current)
# 更新相邻节点的距离和前驱节点
for neighbor, weight in graph[current].items():
new_distance = distance[current] + weight
if new_distance < distance[neighbor]:
distance[neighbor] = new_distance
predecessor[neighbor] = current
return distance, predecessor
# 创建有向图
G = nx.DiGraph()
G.add_edges_from([('A', 'B', {'weight': 5}), ('A', 'D', {'weight': 9}), ('A', 'E', {'weight': 2}),
('B', 'C', {'weight': 2}), ('C', 'D', {'weight': 3}), ('D', 'F', {'weight': 2}),
('E', 'F', {'weight': 3}), ('F', 'G', {'weight': 2})])
# 运行Dijkstra算法
distance, predecessor = dijkstra(G, 'A')
# 绘制图形
pos = nx.spring_layout(G)
nx.draw_networkx_nodes(G, pos)
nx.draw_networkx_edges(G, pos)
nx.draw_networkx_labels(G, pos)
# 绘制最短路径
path = ['G']
while predecessor[path[-1]] is not None:
path.append(predecessor[path[-1]])
path.reverse()
edge_labels = {}
for i in range(len(path)-1):
edge_labels[(path[i], path[i+1])] = distance[path[i+1]] - distance[path[i]]
nx.draw_networkx_edge_labels(G, pos, edge_labels=edge_labels, font_size=8)
nx.draw_networkx_edges(G, pos, edgelist=[(path[i], path[i+1]) for i in range(len(path)-1)], edge_color='r', width=2)
plt.axis('off')
plt.show()
```
该代码将创建一个有向图,并使用Dijkstra算法计算从节点A到其他节点的最短路径,并将最短路径绘制为红色。输出的图形如下所示:

阅读全文
相关推荐
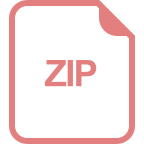

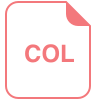
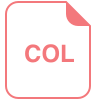
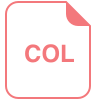


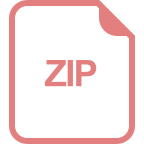
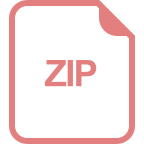
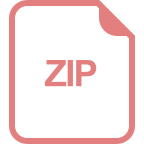
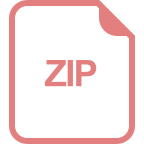
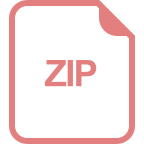
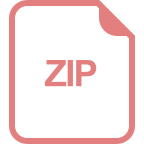
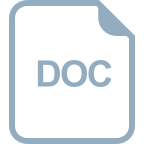
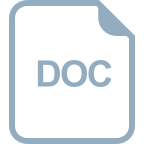
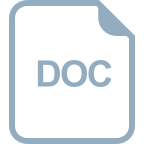