已知y依赖于x,数据如下: x 0.0 0.5 1.0 1.5 1.9 2.5 3.0 3.5 4.0 4.5 5.0 5.5 6.0 6.6 7.0 7.6 8.5 9.0 10 y 1.0 0.9 0.7 1.5 2.0 2.4 3.2 2.0 2.7 3.5 1.0 4.0 3.6 2.7 5.7 4.6 6.0 6.8 7.3 用内置函数fit求其1次、3次、5次拟合多项式,并分析误差。
时间: 2023-06-05 14:09:02 浏览: 236
首先需要导入numpy和matplotlib模块:
```
import numpy as np
import matplotlib.pyplot as plt
```
然后将数据转换为numpy数组:
```
x = np.array([0.0, 0.5, 1.0, 1.5, 1.9, 2.5, 3.0, 3.5, 4.0, 4.5, 5.0, 5.5, 6.0, 6.6, 7.0, 7.6, 8.5, 9.0, 10])
y = np.array([1.0, 0.9, 0.7, 1.5, 2.0, 2.4, 3.2, 2.0, 2.7, 3.5, 1.0, 4.0, 3.6, 2.7, 5.7, 4.6, 6.0, 6.8, 7.3])
```
1次拟合多项式:
```
p1 = np.polyfit(x, y, 1)
y1 = np.polyval(p1, x)
```
3次拟合多项式:
```
p3 = np.polyfit(x, y, 3)
y3 = np.polyval(p3, x)
```
5次拟合多项式:
```
p5 = np.polyfit(x, y, 5)
y5 = np.polyval(p5, x)
```
计算误差:
```
error1 = np.sum((y - y1) ** 2)
error3 = np.sum((y - y3) ** 2)
error5 = np.sum((y - y5) ** 2)
```
将结果绘制成图表:
```
plt.plot(x, y, 'o')
plt.plot(x, y1, label='1次拟合')
plt.plot(x, y3, label='3次拟合')
plt.plot(x, y5, label='5次拟合')
plt.legend()
plt.show()
```
最后输出每种拟合的误差:
```
print("1次拟合误差:", error1)
print("3次拟合误差:", error3)
print("5次拟合误差:", error5)
```
完整代码如下:
```
import numpy as np
import matplotlib.pyplot as plt
# 数据
x = np.array([0.0, 0.5, 1.0, 1.5, 1.9, 2.5, 3.0, 3.5, 4.0, 4.5, 5.0, 5.5, 6.0, 6.6, 7.0, 7.6, 8.5, 9.0, 10])
y = np.array([1.0, 0.9, 0.7, 1.5, 2.0, 2.4, 3.2, 2.0, 2.7, 3.5, 1.0, 4.0, 3.6, 2.7, 5.7, 4.6, 6.0, 6.8, 7.3])
# 拟合
p1 = np.polyfit(x, y, 1)
y1 = np.polyval(p1, x)
p3 = np.polyfit(x, y, 3)
y3 = np.polyval(p3, x)
p5 = np.polyfit(x, y, 5)
y5 = np.polyval(p5, x)
# 计算误差
error1 = np.sum((y - y1) ** 2)
error3 = np.sum((y - y3) ** 2)
error5 = np.sum((y - y5) ** 2)
# 绘图
plt.plot(x, y, 'o')
plt.plot(x, y1, label='1次拟合')
plt.plot(x, y3, label='3次拟合')
plt.plot(x, y5, label='5次拟合')
plt.legend()
plt.show()
# 输出误差
print("1次拟合误差:", error1)
print("3次拟合误差:", error3)
print("5次拟合误差:", error5)
```
阅读全文
相关推荐
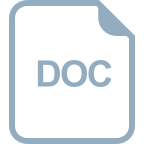
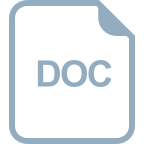
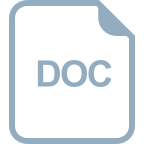
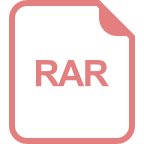
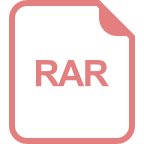
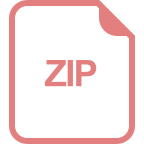
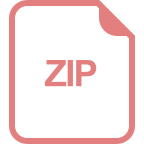
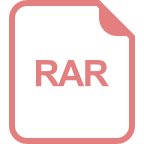
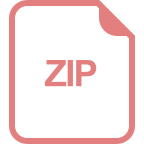
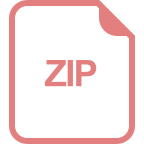
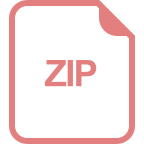
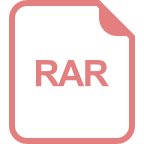
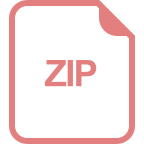
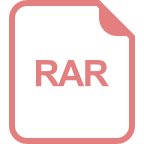
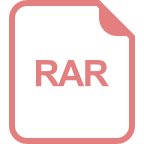
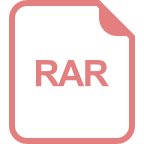
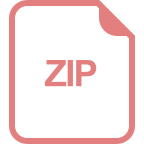
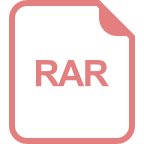
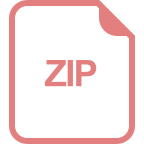