用不同的类去实现一个贪吃蛇游戏的代码
时间: 2024-03-23 22:42:05 浏览: 97
好的,下面是两个不同的类实现贪吃蛇游戏的代码:
第一个类是贪吃蛇类(Snake):
```python
class Snake:
def __init__(self, x, y):
self.x = x
self.y = y
self.body = [(x, y), (x-1, y), (x-2, y)]
self.direction = "right"
def move(self):
if self.direction == "right":
self.x += 1
elif self.direction == "left":
self.x -= 1
elif self.direction == "up":
self.y -= 1
elif self.direction == "down":
self.y += 1
self.body.insert(0, (self.x, self.y))
self.body.pop()
def change_direction(self, direction):
self.direction = direction
def get_head(self):
return self.body[0]
def get_body(self):
return self.body[1:]
```
第二个类是游戏类(Game):
```python
import random
class Game:
def __init__(self, width, height):
self.width = width
self.height = height
self.snake = Snake(width//2, height//2)
self.food = self.generate_food()
self.score = 0
def generate_food(self):
x = random.randint(0, self.width-1)
y = random.randint(0, self.height-1)
while (x, y) in self.snake.get_body() or (x, y) == self.snake.get_head():
x = random.randint(0, self.width-1)
y = random.randint(0, self.height-1)
return (x, y)
def update(self):
self.snake.move()
if self.snake.get_head() == self.food:
self.snake.body.append(self.snake.body[-1])
self.food = self.generate_food()
self.score += 1
elif self.snake.get_head()[0] < 0 or self.snake.get_head()[0] >= self.width or \
self.snake.get_head()[1] < 0 or self.snake.get_head()[1] >= self.height or \
self.snake.get_head() in self.snake.get_body():
return False
return True
```
以上是两个类实现贪吃蛇游戏的代码。
阅读全文
相关推荐
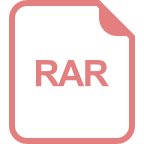
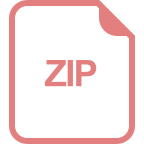
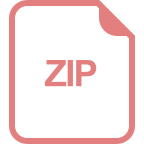
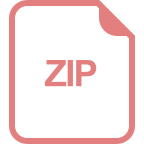
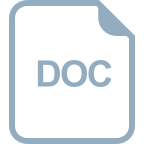
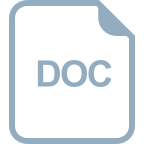
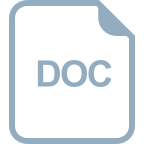
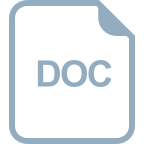
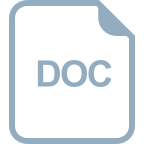
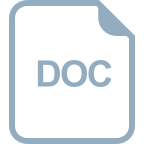
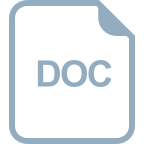
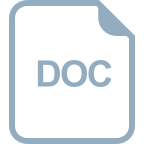
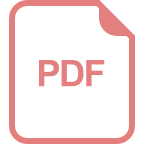
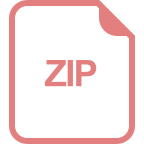
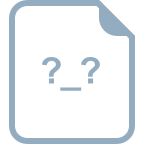