java中创建txt文件的索引
时间: 2023-08-05 08:18:39 浏览: 54
在Java中创建txt文件可以使用FileWriter类。下面是一个简单的示例代码,它可以创建一个名为“example.txt”的文本文件,并向其中写入一些文本:
```java
import java.io.FileWriter;
import java.io.IOException;
public class CreateTextFile {
public static void main(String[] args) {
try {
FileWriter writer = new FileWriter("example.txt");
writer.write("This is some text in the file.");
writer.close();
System.out.println("File created successfully.");
} catch (IOException e) {
System.out.println("An error occurred while creating the file.");
e.printStackTrace();
}
}
}
```
要创建一个文件索引,可以使用Java中的Lucene搜索引擎库。Lucene可以在文本文件中创建索引,以便可以快速搜索和检索文件内容。以下是一个示例代码,它可以使用Lucene创建一个名为“exampleIndex”的索引,并将“example.txt”文件的内容添加到索引中:
```java
import org.apache.lucene.analysis.standard.StandardAnalyzer;
import org.apache.lucene.document.Document;
import org.apache.lucene.document.Field;
import org.apache.lucene.index.IndexWriter;
import org.apache.lucene.store.Directory;
import org.apache.lucene.store.FSDirectory;
import java.io.File;
import java.io.FileReader;
import java.io.IOException;
public class CreateIndex {
public static void main(String[] args) {
try {
// 创建一个目录来存储索引
Directory directory = FSDirectory.open(new File("exampleIndex").toPath());
// 创建一个IndexWriter来写入索引
IndexWriter writer = new IndexWriter(directory, new IndexWriterConfig(new StandardAnalyzer()));
// 创建一个文档对象,将文本文件中的内容添加到文档对象中
Document doc = new Document();
Field contentField = new TextField("content", new FileReader("example.txt"));
doc.add(contentField);
// 将文档对象添加到索引中
writer.addDocument(doc);
// 关闭IndexWriter
writer.close();
System.out.println("Index created successfully.");
} catch (IOException e) {
System.out.println("An error occurred while creating the index.");
e.printStackTrace();
}
}
}
```
请注意,以上代码只是一个简单的示例,您可能需要根据您的具体需求进行修改。
相关推荐
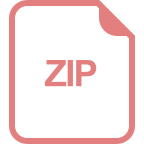
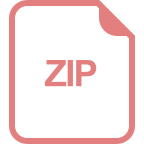
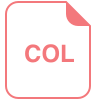
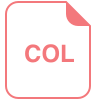
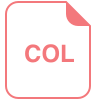
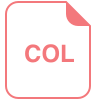
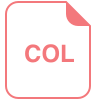









