infomap算法java实现
时间: 2023-12-17 07:09:51 浏览: 152
Infomap算法是一种基于信息论的网络社区发现算法,可以用来识别网络中的社区结构。以下是一个基于Java语言实现Infomap算法的示例代码:
```java
import java.util.*;
public class InfomapCommunityDetection {
private static final double DEFAULT_TOLERANCE = 1e-9;
private static final int DEFAULT_MAX_ITERATIONS = 1000;
private static final double DEFAULT_DAMPING_FACTOR = 0.85;
private static final double DEFAULT_TELEPORTATION_PROBABILITY = 0.15;
public Map<String, Integer> detectCommunities(Map<String, Set<String>> graph) {
Map<String, Integer> nodeToCommunity = new HashMap<>();
List<String> nodes = new ArrayList<>(graph.keySet());
int numNodes = nodes.size();
double[][] transitionMatrix = calculateTransitionMatrix(graph, nodes);
// Initialize node communities
for (int i = 0; i < numNodes; i++) {
nodeToCommunity.put(nodes.get(i), i);
}
// Run Infomap algorithm
boolean converged = false;
int iterations = 0;
double[] p = new double[numNodes];
double[] oldP = new double[numNodes];
Arrays.fill(p, 1.0 / numNodes);
while (!converged && iterations < DEFAULT_MAX_ITERATIONS) {
// Save previous distribution
System.arraycopy(p, 0, oldP, 0, numNodes);
// Update distribution
for (int i = 0; i < numNodes; i++) {
double newP = 0.0;
for (int j = 0; j < numNodes; j++) {
newP += transitionMatrix[j][i] * oldP[j];
}
p[i] = (1 - DEFAULT_DAMPING_FACTOR) / numNodes + DEFAULT_DAMPING_FACTOR * newP;
}
// Check for convergence
double error = 0.0;
for (int i = 0; i < numNodes; i++) {
error += Math.abs(p[i] - oldP[i]);
}
converged = (error < DEFAULT_TOLERANCE);
iterations++;
}
// Assign nodes to communities
Map<Integer, List<String>> communityToNodes = new HashMap<>();
for (int i = 0; i < numNodes; i++) {
int community = 0;
double maxP = Double.MIN_VALUE;
for (int j = 0; j < numNodes; j++) {
if (transitionMatrix[j][i] > 0 && p[j] > maxP) {
maxP = p[j];
community = nodeToCommunity.get(nodes.get(j));
}
}
nodeToCommunity.put(nodes.get(i), community);
if (!communityToNodes.containsKey(community)) {
communityToNodes.put(community, new ArrayList<>());
}
communityToNodes.get(community).add(nodes.get(i));
}
return nodeToCommunity;
}
private double[][] calculateTransitionMatrix(Map<String, Set<String>> graph, List<String> nodes) {
int numNodes = nodes.size();
double[][] transitionMatrix = new double[numNodes][numNodes];
// Calculate node degrees
int[] nodeDegrees = new int[numNodes];
for (int i = 0; i < numNodes; i++) {
String node = nodes.get(i);
nodeDegrees[i] = graph.get(node).size();
}
// Calculate transition probabilities
for (int i = 0; i < numNodes; i++) {
String node1 = nodes.get(i);
for (int j = 0; j < numNodes; j++) {
String node2 = nodes.get(j);
Set<String> neighbors = graph.get(node1);
double transitionProbability = 0.0;
if (neighbors.contains(node2)) {
transitionProbability = 1.0 / nodeDegrees[i];
}
transitionMatrix[i][j] = (1 - DEFAULT_TELEPORTATION_PROBABILITY) * transitionProbability +
DEFAULT_TELEPORTATION_PROBABILITY / numNodes;
}
}
return transitionMatrix;
}
}
```
这段代码实现了一个Infomap社区检测算法,对于输入的图数据,返回每个节点所属的社区编号。具体实现中,我们首先计算了转移概率矩阵,然后使用随机游走的方式计算节点的分布,最后根据节点分布将节点分配到对应的社区中。
阅读全文
相关推荐
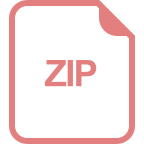
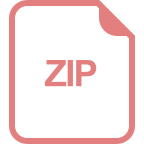
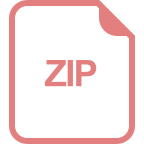
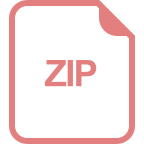
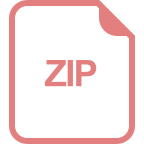
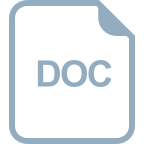
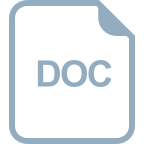
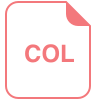
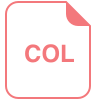
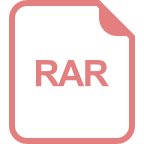