使用大华SDK对接视频监控实时播放和回放示例
时间: 2023-08-08 08:06:09 浏览: 117
以下是一个使用大华SDK对接视频监控实时播放和回放的示例,供参考:
```python
import time
import sys
import os
from ctypes import *
from datetime import datetime
import threading
# 大华SDK库文件路径
SDK_PATH = 'C:\\dahua\\SDK\\DHNetSDK.dll'
# 初始化SDK
NET_DVR_Init = windll.DHNetSDK.NET_DVR_Init
NET_DVR_Init.argtypes = None
NET_DVR_Init.restype = c_bool
NET_DVR_Init()
# 登录设备
NET_DVR_Login_V40 = windll.DHNetSDK.NET_DVR_Login_V40
NET_DVR_Login_V40.argtypes = [POINTER(c_int), POINTER(wchar_t), POINTER(c_int), POINTER(wchar_t), \
POINTER(wchar_t), POINTER(c_int), POINTER(c_int), POINTER(NET_DVR_DEVICEINFO_V40)]
NET_DVR_Login_V40.restype = c_long
lUserID = c_long(-1)
deviceIP = '192.168.1.64'
devicePort = 8000
userName = 'admin'
password = 'admin123'
DeviceInfo = NET_DVR_DEVICEINFO_V40()
ret = NET_DVR_Login_V40(byref(lUserID), deviceIP.encode('utf-8'), devicePort, userName.encode('utf-8'), \
password.encode('utf-8'), None, None, byref(DeviceInfo))
if ret < 0:
print('登录失败,错误码:{}'.format(NET_DVR_GetLastError()))
else:
print('登录成功')
# 实时预览
NET_DVR_RealPlay_V40 = windll.DHNetSDK.NET_DVR_RealPlay_V40
NET_DVR_RealPlay_V40.argtypes = [c_long, POINTER(NET_DVR_PREVIEWINFO), c_void_p, c_void_p]
NET_DVR_RealPlay_V40.restype = c_long
NET_DVR_PREVIEWINFO = struct_net_dvr_previewinfo()
class struct_net_dvr_previewinfo(Structure):
_fields_ = [('lChannel', c_long),
('dwStreamType', c_ulong),
('dwLinkMode', c_ulong),
('hPlayWnd', c_void_p),
('bBlocked', c_bool),
('bPassbackRecord', c_bool),
('byPreviewMode', c_byte),
('byProtoType', c_byte * 3),
('byRes', c_byte * 2),
('union', c_ulong * 2)]
lRealPlayHandle = c_long(-1)
PreviewInfo = NET_DVR_PREVIEWINFO()
PreviewInfo.lChannel = 1
PreviewInfo.dwStreamType = 0
PreviewInfo.dwLinkMode = 0
PreviewInfo.hPlayWnd = None
PreviewInfo.bBlocked = 1
lRealPlayHandle = NET_DVR_RealPlay_V40(lUserID, byref(PreviewInfo), None, None)
if lRealPlayHandle < 0:
print('实时预览失败,错误码:{}'.format(NET_DVR_GetLastError()))
else:
print('实时预览成功')
# 回放
NET_DVR_FindFile_V30 = windll.DHNetSDK.NET_DVR_FindFile_V30
NET_DVR_FindFile_V30.argtypes = [c_long, POINTER(NET_DVR_FILECOND),]
NET_DVR_FindFile_V30.restype = c_long
NET_DVR_FILECOND = struct_net_dvr_filecond()
class struct_net_dvr_filecond(Structure):
_fields_ = [('lChannel', c_long),
('dwFileType', c_ulong),
('dwIsLocked', c_ulong),
('dwUseCardNo', c_ulong),
('struStartTime', NET_DVR_TIME),
('struStopTime', NET_DVR_TIME),
('sCardNumber', c_byte * 32),
('dwFileSize', c_ulong)]
NET_DVR_TIME = struct_net_dvr_time()
class struct_net_dvr_time(Structure):
_fields_ = [('dwYear', c_ulong),
('dwMonth', c_ulong),
('dwDay', c_ulong),
('dwHour', c_ulong),
('dwMinute', c_ulong),
('dwSecond', c_ulong)]
NET_DVR_FindNextFile_V30 = windll.DHNetSDK.NET_DVR_FindNextFile_V30
NET_DVR_FindNextFile_V30.argtypes = [c_long, POINTER(NET_DVR_FINDDATA_V30)]
NET_DVR_FindNextFile_V30.restype = c_bool
NET_DVR_FINDDATA_V30 = struct_net_dvr_finddata_v30()
class struct_net_dvr_finddata_v30(Structure):
_fields_ = [('sFileName', c_byte * 100),
('struStartTime', NET_DVR_TIME),
('struStopTime', NET_DVR_TIME),
('dwFileSize', c_ulong),
('sCardNum', c_byte * 32),
('byLocked', c_byte),
('byFileType', c_byte),
('byQuickSearch', c_byte),
('byRes', c_byte * 2)]
NET_DVR_PlayBackByTime_V40 = windll.DHNetSDK.NET_DVR_PlayBackByTime_V40
NET_DVR_PlayBackByTime_V40.argtypes = [c_long, POINTER(NET_DVR_VOD_PARA), c_void_p]
NET_DVR_PlayBackByTime_V40.restype = c_long
NET_DVR_VOD_PARA = struct_net_dvr_vod_para()
class struct_net_dvr_vod_para(Structure):
_fields_ = [('dwSize', c_ulong),
('struIDInfo', NET_DVR_STREAM_INFO),
('hWnd', c_void_p),
('lpUserData', c_void_p),
('struBeginTime', NET_DVR_TIME),
('struEndTime', NET_DVR_TIME),
('byDrawFrame', c_byte),
('byVolumeType', c_byte),
('byVolumeNum', c_byte),
('byStreamType', c_byte),
('byAudioType', c_byte),
('byVideoEncType', c_byte),
('byAudioEncType', c_byte),
('byVideoResolution', c_byte),
('byNetType', c_byte),
('dwEngineVersion', c_ulong),
('bySupportStreamEncrypt', c_byte),
('byRes', c_byte * 7)]
NET_DVR_STREAM_INFO = struct_net_dvr_stream_info()
class struct_net_dvr_stream_info(Structure):
_fields_ = [('dwSize', c_ulong),
('byID', c_byte * 48),
('byRes', c_byte * 4),
('dwChannel', c_ulong),
('byRes1', c_byte * 16)]
lPlaybackHandle = c_long(-1)
FileCond = NET_DVR_FILECOND()
FileCond.lChannel = 1
FileCond.dwFileType = 0xff
FileCond.struStartTime.dwYear = 2021
FileCond.struStartTime.dwMonth = 7
FileCond.struStartTime.dwDay = 1
FileCond.struStartTime.dwHour = 0
FileCond.struStartTime.dwMinute = 0
FileCond.struStartTime.dwSecond = 0
FileCond.struStopTime.dwYear = 2021
FileCond.struStopTime.dwMonth = 7
FileCond.struStopTime.dwDay = 1
FileCond.struStopTime.dwHour = 23
FileCond.struStopTime.dwMinute = 59
FileCond.struStopTime.dwSecond = 59
FileHandle = NET_DVR_FindFile_V30(lUserID, byref(FileCond))
if FileHandle >= 0:
FindData = NET_DVR_FINDDATA_V30()
while NET_DVR_FindNextFile_V30(FileHandle, byref(FindData)):
PlaybackInfo = NET_DVR_VOD_PARA()
PlaybackInfo.struIDInfo.dwChannel = FileCond.lChannel
PlaybackInfo.struBeginTime = FindData.struStartTime
PlaybackInfo.struEndTime = FindData.struStopTime
lPlaybackHandle = NET_DVR_PlayBackByTime_V40(lUserID, byref(PlaybackInfo), None)
if lPlaybackHandle < 0:
print('回放失败,错误码:{}'.format(NET_DVR_GetLastError()))
else:
print('回放成功')
time.sleep(10)
NET_DVR_StopPlayBack = windll.DHNetSDK.NET_DVR_StopPlayBack
NET_DVR_StopPlayBack.argtypes = [c_long]
NET_DVR_StopPlayBack.restype = c_bool
NET_DVR_StopPlayBack(lPlaybackHandle)
NET_DVR_FindClose = windll.DHNetSDK.NET_DVR_FindClose
NET_DVR_FindClose.argtypes = [c_long]
NET_DVR_FindClose(FileHandle)
# 释放SDK资源
NET_DVR_Logout = windll.DHNetSDK.NET_DVR_Logout
NET_DVR_Logout.argtypes = [c_long]
NET_DVR_Logout.restype = c_bool
NET_DVR_Logout(lUserID)
NET_DVR_Cleanup = windll.DHNetSDK.NET_DVR_Cleanup
NET_DVR_Cleanup.argtypes = None
NET_DVR_Cleanup.restype = c_bool
NET_DVR_Cleanup()
```
需要注意的是,以上示例代码中使用的`NET_DVR_PREVIEWINFO`和`NET_DVR_VOD_PARA`结构体以及相关的字段定义均来自于大华SDK文档,具体可根据实际应用场景进行调整。此外,因为回放需要先查找可用的录像文件,因此在回放示例中还使用了`NET_DVR_FindFile_V30`和`NET_DVR_FindNextFile_V30`函数来查找录像文件。
相关推荐
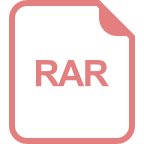














