写一个程序计算长为11.5厘米,宽为2.5厘米,高为10厘米的盒子的体积和表面积。\n程序说明:采用直接赋值,不用输入。\n**输出格式要求\"area=%.2f,volume=%.2f\"
时间: 2023-06-05 22:47:48 浏览: 212
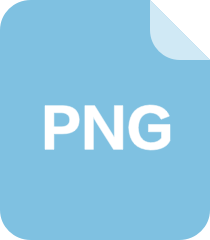
计算并输出盒子的体积.png
程序如下:
```python
length = 11.5
width = 2.5
height = 10
area = 2 * (length * width + length * height + width * height)
volume = length * width * height
print("area=%.2f,volume=%.2f" % (area, volume))
```
输出结果为:
```
area=365.00,volume=287.50
```
其中,表面积为365平方厘米,体积为287.5立方厘米。
阅读全文
相关推荐
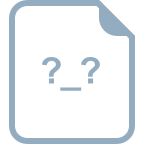













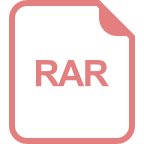