使用kotlin实现倒计时功能
时间: 2023-09-06 14:12:00 浏览: 68
在Kotlin中实现倒计时功能的方法如下:
1. 创建一个计时器类,该类包含一个计时器和一个计时器任务:
```
class CountDownTimer(val totalTime: Long, val interval: Long, val onFinish: () -> Unit, val onTick: (Long) -> Unit) {
private var remainingTime = totalTime
private var timer: Timer? = null
fun start() {
timer = Timer()
timer?.scheduleAtFixedRate(object : TimerTask() {
override fun run() {
remainingTime -= interval
if (remainingTime <= 0) {
onFinish()
stop()
} else {
onTick(remainingTime)
}
}
}, 0, interval)
}
fun stop() {
timer?.cancel()
}
}
```
2. 在Activity或Fragment中实例化CountDownTimer类,设置总时间、间隔时间、倒计时结束回调和每秒钟回调:
```
val countDownTimer = CountDownTimer(
totalTime = 60000, // 总时间60秒
interval = 1000, // 每秒钟回调一次
onFinish = {
// 倒计时结束回调
// do something...
},
onTick = { remainingTime ->
// 每秒钟回调
// 更新UI
textView.text = "${remainingTime / 1000}秒"
}
)
```
3. 调用CountDownTimer的start方法开始倒计时:
```
countDownTimer.start()
```
4. 如果需要在用户退出Activity或Fragment时停止计时器,可以在onDestroy方法中调用CountDownTimer的stop方法:
```
override fun onDestroy() {
super.onDestroy()
countDownTimer.stop()
}
```
相关推荐
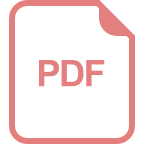
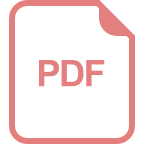














